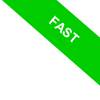
Methods in Java
In Java, a method is a block of code designed to carry out a specific task or perform a calculation. Similar to functions in other programming languages, methods in Java are always associated with a class or an object.
What are they used for? Methods are crucial for organizing code, making it more readable and facilitating the reuse of common functionalities across different parts of a program. They serve as powerful tools for structuring code, promoting reuse, and achieving abstraction, which in turn makes the code more maintainable and modular.
Think of methods in Java like tools in a toolbox: each tool is crafted to perform a specific job, making the work more efficient. When building a program, using the appropriate methods is like using the right tools to complete a complex project efficiently and with precision.
The Structure of Methods
A method in Java consists of several key elements. Let’s examine a simple example:
public class Example {
// Method definition
public int add(int a, int b) {
int result = a + b; // Method body
return result; // Returns an integer value
}
public static void main(String[] args) {
Example example = new Example();
int result = example.add(5, 3);
System.out.println("The sum is: " + result);
}
}
In this program, there are two methods: the add() method and the main() method:
The main() method is particularly important because it’s a special method that serves as the entry point for the program's execution. When you run the program, the Java Virtual Machine automatically looks for and executes the code block contained in the main() method.
The add() method, on the other hand, is executed only when it is explicitly called by the program.
Let’s break down the main components of a method:
- Access Modifiers
These determine the method's visibility from other classes.
- `public`: The method is accessible from any other class.
- `private`: The method is accessible only within the class in which it is defined.
- `protected`: The method is accessible by classes in the same package or by subclasses. - Return Type
Specifies the type of data the method will return. If the method does not return any value, the `void` keyword is used. - Method Name
Must be a valid identifier, following the camelCase convention. - Parameters
These are optional. If present, they are listed within parentheses after the method name. Each parameter requires a type and a name. - Method Body
This is the block of code enclosed in curly braces `{ }` that contains the operations of the method. - `return` Keyword
Used to return a value from the method if the return type is not `void`. When executed, it immediately terminates the method's execution.
Types of Methods
Instance Methods
These are tied to a specific instance (object) of a class. To call them, you must first create an object of the class.
public class Example {
public void greet() {
System.out.println("Hello!");
}
}
public static void main(String[] args) {
Example example = new Example();
example.greet(); // Call to instance method
}
Static Methods
These are associated with the class itself and can be called directly using the class name, without needing to create an object.
public class Example {
public static void greet() {
System.out.println("Hello!");
}
}
public static void main(String[] args) {
Example.greet(); // Call to static method
}
Constructor Methods
These are special methods used to initialize new objects of a class. A constructor shares the same name as the class and does not have a return type.
public class Example {
private int value;
// Constructor
public Example(int val) {
value = val;
}
public int getValue() {
return value;
}
}
public static void main(String[] args) {
Example example = new Example(10);
System.out.println("Value: " + example.getValue());
}
Abstract Methods
Declared in abstract classes, these methods do not have a body. Concrete subclasses must provide an implementation for these methods.
abstract class Shape {
abstract void draw(); // Abstract method
}
class Circle extends Shape {
void draw() {
System.out.println("Draw a circle");
}
}
Final Methods
A method declared as `final` cannot be overridden by a subclass.
Methods as a Way of Thinking
In addition to being a fundamental technical element, methods in Java also teach us valuable lessons about modularity and organization, both in coding and in life.
Learning to break down complex problems into smaller, more manageable components, much like we do with methods, helps us simplify the challenges we face every day. It serves as a reminder that just as a method can be reused in different contexts, our experiences and skills can be applied across various aspects of our lives.
In a way, programming is not just a technical activity; it's an exercise in logical thinking, organization, and creativity—qualities that are fundamentally human.
I hope this introduction to Java methods has been helpful to you.