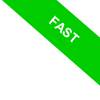
Main() Method in Java
What is the `main()` method in Java?
The `main()` method in Java serves as the starting point of any program. It's where the program begins its execution.
When you run a Java program, the Java Virtual Machine (JVM) looks for the `main()` method in the specified class and starts executing the code within it.
Why is the `main()` method important? The `main()` method is essential because it directs the JVM where to start executing the program. Without it, the JVM wouldn't know where to begin. It's akin to the starting line of a race: without a clear start, no one would know when to begin running.
For the JVM to recognize the `main()` method, it needs to have the following signature:
public static void main(String[] args) {
// Your program's code goes here
}
Here's a breakdown of the `main()` method signature:
- public
This keyword indicates that the method is accessible from anywhere in the program. Since it serves as the entry point, it must be public so the JVM can invoke it. - static
This means the method belongs to the class itself rather than to a specific instance. This is necessary because the JVM must be able to call the method without needing to create an instance of the class. - void
This specifies that the method doesn't return any value. Once the `main()` method completes, the program terminates without returning a result. - String[] args
This parameter represents an array of strings containing any command-line arguments passed to the program. For instance, if you run the program with `java ClassName arg1 arg2`, the `args` array will contain `{"arg1", "arg2"}`.
Practical Example
Let's look at a simple Java program:
public class HelloWorld {
public static void main(String[] args) {
// Prints "Hello, world!" to the console
System.out.println("Hello, world!");
// Prints the number of arguments passed to the program
System.out.println("Number of arguments: " + args.length);
}
}
Now run this program without passing any arguments:
java HelloWorld
You'll see this output:
Hello, world!
Number of arguments: 0
Next, run the program with two arguments:
java HelloWorld one two
This time, the output will be:
Hello, world!
Number of arguments: 2
Using Command-Line Arguments
Command-line arguments provide a way to pass information to your program at the time of execution.
In the `main()` method, these arguments can be accessed through the `String[] args` array.
Here's an example of how to use them:
public class GreetUser {
public static void main(String[] args) {
if (args.length > 0) {
System.out.println("Hello, " + args[0] + "!");
} else {
System.out.println("Hello, stranger!");
}
}
}
To run this program, type the following in your terminal:
java GreetUser Richard
The output will be:
Hello, Richard!
If you run it without passing any arguments, you'll see:
java GreetUser
Hello, stranger!
In conclusion, the `main()` method is the starting point for any Java application. While its structure is straightforward, it's vital to understand how it works and how to manage command-line arguments effectively.
Understanding these concepts will enable you to develop Java applications more efficiently.
I hope this explanation has helped clarify the `main()` method!