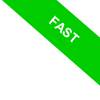
Method Parameters in Java
In Java, parameters are variables that allow a method to receive values from outside.
When you define a method, you can specify a list of parameters that the method will use during its execution.
These parameters are declared in the method definition and can be used within its body to perform various operations.
To put it simply, method parameters are like the fields in a tax form that you need to fill out before submitting it. Each field in the form is a parameter that requires specific information. The method uses this information to perform its task, much like a clerk who processes the form after you've completed it.
Let’s consider a practical example.
Imagine you have a class called `Example`. Inside this class, there’s a method called `sum` that takes two numbers, adds them together, and returns the result.
public class Example {
// Definition of a method that accepts two parameters
public int sum(int a, int b) {
// The method uses the parameters 'a' and 'b' to calculate the sum
return a + b;
}
public static void main(String[] args) {
Example example = new Example();
// Calling the 'sum' method with the values 5 and 3 as arguments
int result = example.sum(5, 3);
System.out.println("The sum is: " + result);
}
}
The `sum` method accepts two parameters, `a` and `b`, which represent the numbers you want to add. When you call this method and pass in 5 and 3, it adds them together and returns 8.
In the `main` method, which is the entry point of the program, you create an instance of the `Example` class.
You then call the `sum` method on this instance, passing 5 and 3 as arguments.
The result of the addition is stored in the `result` variable, and finally, you print the value of `result`, which in this case is 8.
The sum is 8
In summary, this code demonstrates how to create a method that adds two numbers and how to use it within a program to calculate and display the result.
Understanding Parameters
Let’s take a closer look at what constitutes a parameter in Java.
- Type
Each parameter has a specific type (such as `int`, `String`, `double`, etc.) that defines the kind of data the method can accept. - Name
Parameters are identified by a name, which is used within the method to refer to the value passed. This name follows the same rules as variable names. - Passed Value
When you call a method, you can pass values to the parameters. These values, called arguments, are assigned to the corresponding parameters during the method’s execution. - Variable Number of Parameters
Java allows you to define methods with a variable number of parameters using the `...` (three dots) syntax. This feature is useful when you don’t know in advance how many arguments will be passed to the method.
public void printNumbers(int... numbers) {
for (int number : numbers) {
System.out.println(number);
}
}
Additionally, it's important to note that in Java, parameters are passed by value.
This means that the method receives a copy of the value of the argument passed. As a result, any changes made to the parameter inside the method do not affect the original argument.
For example, take a look at this code.
public class Example {
public static void main(String[] args) {
int number = 10;
modifyValue(number);
System.out.println("Original value: " + number); // Prints "Original value: 10"
}
public static void modifyValue(int n) {
n = 20; // Modifies only the local copy
}
}
In this example, the variable `number` holds the value 10.
When you pass `number` to the modifyValue() method, a copy of this value is created. The reference to the original object is not passed.
The `modifyValue()` method changes the copy by setting the variable to 20, but the original `number` variable remains 10.
When you print the original value, you see that it hasn’t changed.
Original value 10
This characteristic of pass-by-value in Java might seem limiting at first, but it actually provides a level of safety: you can be sure that the original variables won’t be accidentally altered by a method that uses them.
It’s like having a backup copy that you can manipulate without worrying about affecting the original.
Method parameters in Java are therefore essential for making methods flexible and reusable, allowing them to operate on different values each time they are called.
There are situations where you might actually want to change the original value, and in those cases, you’ll need to use different strategies, such as working with objects or arrays, which behave a bit differently. It’s important to keep this in mind.