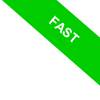
Comments in Java
Writing comments in Java is a skill that can greatly enhance the readability of your code, not just for yourself, but for anyone else who may work with it in the future.
Why are comments important in a program? Comments are parts of the code that the compiler ignores, so they don’t play a role in the program’s execution. Their purpose is to clarify what the code does, explain why it was written in a certain way, and highlight key concepts. This makes future modifications easier and the code more maintainable.
In Java, there are several ways to include comments in your code.
Single-Line Comments (`//`)
These comments are used to explain individual lines of code or small code blocks.
They’re particularly useful for providing quick context or explanations.
// Check if the user meets the minimum age requirement
if (age >= 18) {
System.out.println("Access granted");
}
In this example, the comment succinctly describes the purpose of the condition. Even though the code is straightforward, the comment makes its intent crystal clear.
Block Comments (`/* ... */`)
Block comments, marked by /* ... */, are perfect for explaining larger sections of code or for providing an overview of a function or class.
/*
* This function calculates the factorial of a number.
* The factorial of n is the product of all positive integers less than or equal to n.
* Example: 5! = 5 * 4 * 3 * 2 * 1 = 120
*/
public int factorial(int n) {
if (n == 0) {
return 1; // The factorial of 0 is defined as 1
} else {
return n * factorial(n - 1); // Recursively calculates the factorial
}
}
Here, the comment not only describes the function but also provides a concrete example of its functionality.
This is especially useful for readers who might not be familiar with the concept of a factorial.
By convention, each line in a code block should start with an asterisk "*", ensuring the comment is easily distinguishable and clearly defined.
Javadoc Comments (`/** ... */`)
Javadoc comments /** ... */ are special because they’re used to generate automatic documentation for your code.
They’re placed above classes, methods, or variables and provide a formal description of their functionality.
/**
* This class represents a circle with a specified radius.
* It provides methods to calculate the area and circumference.
*/
public class Circle {
private double radius;
/**
* Constructor for the Circle class.
* @param radius The radius of the circle.
*/
public Circle(double radius) {
this.radius = radius;
}
/**
* Calculates the area of the circle.
* @return The area of the circle.
*/
public double calculateArea() {
return Math.PI * Math.pow(radius, 2);
}
/**
* Calculates the circumference of the circle.
* @return The circumference of the circle.
*/
public double calculateCircumference() {
return 2 * Math.PI * radius;
}
}
In this example, Javadoc comments describe the class and its methods in a structured way.
Using Javadoc syntax, you can also include special tags like `@param` to explain parameters and `@return` to describe the return value.
Similarly, each line in a JavaDoc comment (Java Documentation Comment) should also begin with an asterisk "*" as a standard practice.
What’s the difference between `/* ... */` and `/** ... */` comments? The main difference between `/* ... */` and `/** ... */` comments lies in their purpose and usage.
- /* ... */
A general-purpose block comment, used to explain code sections or add notes. It’s not intended for formal documentation. - /** ... */
A Javadoc comment, used to generate official code documentation. These comments are processed by tools to create readable documentation for developers.
Both types of comments are important, but they serve different purposes: the former for internal and informal notes, the latter for structured and official documentation of the source code.
Practical Tips for Writing Comments
To wrap things up, here are some practical tips for writing effective comments.
- Be clear and concise: Comments should be brief and to the point. Avoid writing long explanations!
- Avoid stating the obvious: You don’t need to comment on every single line of code, especially if it’s self-explanatory. For instance, there’s no need to comment on a line like `int a = 5; // Sets a to 5` unless there’s a specific reason to do so.
- Keep comments up-to-date: If you modify the code, make sure to update the comments as well. There’s nothing worse than a comment that no longer matches the code!
- Use comments to explain the “why”: The code shows *what* the program does, but comments should explain *why* it does it, especially if the logic isn’t immediately obvious.
Remember, writing good comments is a key part of becoming a skilled programmer.
Not only does it improve your ability to understand your own code in the future, but it