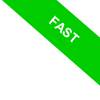
Java Operators
In Java, an operator is a symbol that performs a specific operation on one or more operands (which can be values or variables). Java operators are grouped into several categories:
Arithmetic Operators
Arithmetic operators are used for basic mathematical operations like addition, subtraction, multiplication, and division.
Operator | Description | Example |
---|---|---|
+ | Addition | a + b |
- | Subtraction | a - b |
* | Multiplication | a * b |
/ | Division | a / b |
% | Modulus | a % b |
Assignment Operators
Assignment operators are used to assign values to variables.
Operator | Description | Example |
---|---|---|
= | Simple Assignment | a = 5 |
+= | Add and Assign | a += 5 |
-= | Subtract and Assign | a -= 3 |
*= | Multiply and Assign | a *= 2 |
/= | Divide and Assign | a /= 2 |
%= | Modulus and Assign | a %= 2 |
Relational Operators
Relational operators compare two values and return a boolean result (true
or false
).
Operator | Description | Example |
---|---|---|
== | Equal to | a == b |
!= | Not equal to | a != b |
> | Greater than | a > b |
< | Less than | a < b |
>= | Greater than or equal to | a >= b |
<= | Less than or equal to | a <= b |
Logical Operators
Logical operators combine boolean expressions.
Operator | Description | Example |
---|---|---|
&& | Logical AND | a && b |
|| | Logical OR | a || b |
! | Logical NOT | !a |
Increment and Decrement Operators
These operators increase or decrease the value of a variable by one.
Operator | Description | Example |
---|---|---|
++ | Increment | a++ or ++a |
-- | Decrement | a-- or --a |
Bitwise Operators
These operators work at the bit level on integers.
Operator | Description | Example |
---|---|---|
& | Bitwise AND | a & b |
| | Bitwise OR | a | b |
^ | Bitwise XOR | a ^ b |
~ | Bitwise NOT | ~a |
<< | Left Shift | a << 2 |
>> | Right Shift | a >> 2 |
>>> | Unsigned Right Shift | a >>> 2 |
Ternary Conditional Operator
The ternary operator provides a shorthand way to express a condition.
Operator | Description | Example |
---|---|---|
?: | Ternary Operator | (a > b) ? a : b |
Type Casting Operators
Type casting operators are used to convert one data type into another.
Operator | Description | Example |
---|---|---|
(type) | Explicit Cast | (int) a |
These are the core operators in Java, widely used to manipulate data and control the flow of a program.