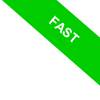
Assignment Operators in Java
In Java (and many other programming languages), an assignment operator is used to assign a value to a variable.
The most common assignment operator is the simple equal sign `=`, which allows us to set a value for a variable.
Here's a straightforward example:
int x = 5;
In this case, we're instructing the program: "Assign the value 5 to the variable `x`."
It's similar to telling the computer: "Place a 5 in the container labeled `x`."
However, assignment operators aren't limited to just this. Java also offers other variations known as compound assignment operators.
Operator | Description | Example |
---|---|---|
= | Simple assignment | a = 5 |
+= | Addition and assignment | a += 5 |
-= | Subtraction and assignment | a -= 3 |
*= | Multiplication and assignment | a *= 2 |
/= | Division and assignment | a /= 2 |
%= | Modulo and assignment | a %= 2 |
Now, let's go through some examples to understand these operators more clearly.
Addition and Assignment Operator
Suppose you have a variable named `balance` that represents the amount of money in your wallet:
int balance = 100;
If you want to add 50 euros to the balance, you can do it in two ways:
balance = balance + 50;
Alternatively, you can use the compound assignment operator `+=`:
balance += 50;
Both methods achieve the same result, but using `+=` is more concise and easier to read.
Now, the value of `balance` is 150.
Multiplication and Assignment Operator
Let's say you want to double the value of your `balance`:
You could use the multiplication operator `*` like this:
balance = balance * 2;
Or, you can use the compound operator `*=` to make it more streamlined:
balance *= 2;
Again, the result is the same, but the second method is much more concise.
Now, `balance` is 300 (if it was 150 before).
Subtraction and Assignment Operator
What if you want to subtract an amount? Suppose you spend 30 euros.
In a traditional way, you would write:
balance = balance - 30;
Using the compound operator `-=`, you can get the same result in a more succinct manner:
balance -= 30;
Now, `balance` will be 270 (if it was 300 before).
This is the advantage of assignment operators: they make common operations much more straightforward.
Division and Assignment Operator
Suppose you want to divide your `balance` by 2:
balance = balance / 2;
You can also use the compound operator `/=` (division and assignment):
balance /= 2;
The final result is the same; the value of `balance` is now halved!
Now, `balance` holds the value 150 (if it was 300 before).
Modulo and Assignment Operator
The `%=` operator is useful when you want to find the remainder of a division.
For example, let's say you have 100 apples and you want to distribute them into groups of 7:
int apples = 100;
apples = apples % 7;
You can achieve the same result using the compound operator `%=`:
int apples = 100;
apples %= 7;
After this operation, `apples` will contain the value 2, which is the remainder when 100 is divided by 7.
Why Use Compound Assignment Operators?
In summary, assignment operators are powerful tools that make our code shorter and more readable.
The best part is that they work with all numeric data types in Java, not just integers. For example, you can use them with `float`, `double`, and even more complex types like `long`.
So, the next time you're writing something like `x = x + y`, remember the compound assignment operators!
They save you time and lines of code, making your program not only cleaner but also easier to maintain.