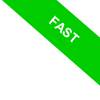
Break Statement in Java
In Java, the `break` statement is quite straightforward, but it’s incredibly useful when you need to exit or stop a loop or a `switch` statement prematurely.
break
Think of `break` as an emergency stop: when the program encounters it, execution halts immediately, and you exit the current loop or block of code.
You can use this statement within loops or conditional structures.
In simple terms, the `break` statement is like a stop command that lets you control when to exit `for` loops, `while` loops, or blocks of code like a `switch` statement. Without it, you could end up executing unnecessary code. In the following sections, we’ll go through a few examples to make everything clearer. It’s an easy feature to use.
Using Break in `for` Loops
Imagine you need to search for a number in an array.
Once you find it, there’s no reason to continue searching, right? This is where `break` comes in.
Here’s an example:
public class Main {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9};
int target = 5;
for (int i = 0; i < numbers.length; i++) {
if (numbers[i] == target) {
System.out.println("Number found: " + numbers[i]);
break; // Exit the loop as soon as the number is found
}
}
}
}
In this example, the `for` loop iterates through each element in the array.
Once it finds the target number, it prints the result and uses `break` to stop the loop immediately.
Number found: 5
Without `break`, the loop would continue running until the end, even though the target number had already been found.
Break in `while` Loops
The concept is the same when it comes to `while` loops.
For instance, let’s say you’re reading numbers from user input until a negative number is entered. In this case, you’d want to stop the loop as soon as that happens:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
while (true) {
// Infinite loop, will stop only with a break
System.out.print("Enter a number (negative number to quit): ");
int number = scanner.nextInt();
if (number < 0) {
break; // Exit the loop if the number is negative
}
System.out.println("You entered: " + number);
}
System.out.println("You entered a negative number. Program terminated.");
}
}
In this case, the `while` loop runs continuously until the user inputs a negative number.
Once that happens, the `break` statement stops the loop, and the program exits.
Enter a number (negative number to quit): 11
You entered: 11
Enter a number (negative number to quit): 3
You entered: 3
Enter a number (negative number to quit): -4
You entered a negative number. Program terminated.
Break in `switch` Statements
Now let’s look at another common use of `break`, inside a `switch` statement.
Here, `break` is crucial to prevent what’s called "fall-through," which occurs when execution passes from one case to the next.
Here’s a practical example:
public class Main {
public static void main(String[] args) {
int day = 3;
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
case 4:
System.out.println("Thursday");
break;
case 5:
System.out.println("Friday");
break;
default:
System.out.println("Weekend!");
}
}
}
In this example, if `day` equals 3, the switch prints "Wednesday" and then stops.
Wednesday
Without `break`, the program would continue executing the remaining cases, printing "Thursday" and "Friday," even though it shouldn't!
What Happens Without `break`?
Let’s remove the `break` statements from the `switch` structure and see what happens.
public class Main {
public static void main(String[] args) {
int day = 3;
switch (day) {
case 1:
System.out.println("Monday");
case 2:
System.out.println("Tuesday");
case 3:
System.out.println("Wednesday");
case 4:
System.out.println("Thursday");
case 5:
System.out.println("Friday");
default:
System.out.println("Weekend!");
}
}
}
If `day` equals 3, the program will print "Wednesday", "Thursday", "Friday", and "Weekend!".
This happens because the execution doesn’t stop after reaching the correct case.
Wednesday
Thursday
Friday
Weekend!
Chances are, this isn’t the outcome you intended.
Therefore, the `break` statement is necessary to stop execution after finding the correct case.
At this point, you should have a clear understanding of how and why to use the `break` statement in Java.