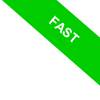
Ternary Conditional Operator in Java
The ternary conditional operator in Java is a shorthand that lets you make decisions in a single line, without needing a full `if-else` block. Think of it as a quick decision: "If this is true, do this; otherwise, do that." Simple, right?
How does the ternary operator work?
In essence, it's a compact form of `if-else`. Here's the syntax:
condition ? value_if_true : value_if_false;
Let’s break it down:
- Condition: This is the expression you're evaluating. It should return either `true` or `false`, such as a comparison or a check.
- Value_if_true: The value returned if the condition evaluates to `true`.
- Value_if_false: The value returned if the condition evaluates to `false`.
A practical example
Imagine you have a number and you want to check whether it's even or odd.
Traditionally, you'd use an `if-else` block like this:
int number = 5;
String result;
if (number % 2 == 0) {
result = "even";
} else {
result = "odd";
}
System.out.println("The number is " + result);
With the ternary operator, however, you can achieve the same result in fewer lines and with a bit more elegance:
int number = 5;
String result = (number % 2 == 0) ? "even" : "odd";
System.out.println("The number is " + result);
See? It all fits into a single line!
The ternary operator checks if `number % 2 == 0`. If it’s true, it returns `"even"`, otherwise, it returns `"odd"`.
Why use it? Well, sometimes your code can get bogged down with too many `if-else` blocks. The ternary operator is a useful shortcut for quick, simple decisions. It’s ideal when you’re working with just two possibilities (true or false) and you want to keep your code concise and readable.
A classic example is finding the larger of two numbers.
Let’s say you want to compare two numbers to determine which is greater. Here's a straightforward example:
int a = 10;
int b = 20;
int max = (a > b) ? a : b;
System.out.println("The larger number is " + max);
Here, you're comparing `a` and `b`.
If `a` is greater than `b`, the variable `max` will take the value of `a`; otherwise, it will take the value of `b`.
Be careful not to overuse it
If the logic in your condition gets too complicated, using the ternary operator can make your code harder to follow. For example:
String result = (a > b) ? ((a > c) ? "a is the largest" : "c is the largest") : ((b > c) ? "b is the largest" : "c is the largest");
Did you catch all that? I didn’t, not at first glance! The code becomes difficult to read. So, if you go overboard with ternary operators, it can just add confusion. In such cases, it's better to stick with a clear and structured `if-else` block.
In conclusion, the ternary conditional operator is a sleek, efficient shortcut that can make your code more compact and easier to read... as long as you use it for straightforward decisions.
However, if the logic becomes complex, it’s probably best to go back to the well-organized and easily understandable `if-else`.
Hopefully, the ternary operator in Java now seems less mysterious and more practical to you.