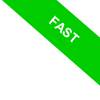
For Loop in Java
The for loop is a core feature of Java that allows you to repeat an action or block of code a specific number of times.
Think of it as an automated machine performing the same action over and over until it says, "Okay, I’m done!" In a for loop, you specify exactly how many times the task should be performed, and it faithfully follows your instructions.
It’s useful for counting, iterating over data, or performing multiple operations automatically, saving you time and effort.
How a for loop works
A for loop in Java follows a simple structure:
for (initialization; condition; update) {
// code to execute
}
Let’s break it down, as each part plays a crucial role:
- Initialization: This is where you tell the loop where to begin. Typically, you initialize a counter variable, which increments after each iteration. Commonly, we use a counter like `int i = 0;`, signaling the loop to start with `i = 0`. Of course, any variable name will work, int t = 0;` or `int k = 0;` are equally valid.
- Condition: This defines when the loop should stop. As long as the condition remains true, the loop continues executing. For instance, `i < 5` means: "Keep looping as long as `i` is less than 5."
- Update: Here, you define how to modify the counter variable at the end of each iteration. It’s usually an increment like `i++`, which means "Increase `i` by one each time." However, you can also decrement it or apply any arithmetic operation that changes the counter.
Remember, "loop" and "iteration" aren’t the same thing. A "loop" refers to repeating a block of code multiple times, whereas an "iteration" is a single pass through the loop. In other words, an iteration is one cycle, and a loop consists of one or more cycles.
Now, let’s look at a practical example!
This code prints the numbers from 0 to 4:
for (int i = 0; i < 5; i++) {
System.out.println("The number is: " + i);
}
Here’s what’s happening: the loop starts with `i = 0`.
Each time the loop runs, it checks the condition (i < 5) to see if `i` is less than 5.
- If the condition is true, Java executes the code inside the braces, increases the counter `i` by 1 (`i++`), and goes back to check the condition again.
- If the condition is false, Java exits the loop.
The output will be:
The number is: 0
The number is: 1
The number is: 2
The number is: 3
The number is: 4
But why stop there? Let’s look at another example!
Suppose you want to calculate the sum of all numbers from 1 to 10.
int sum = 0;
for (int i = 1; i <= 10; i++) {
sum += i; // Adds the value of i to the sum variable
}
System.out.println("The sum is: " + sum);
In this case, the loop starts at `i = 1` and continues as long as `i` is less than or equal to 10.
After each iteration, the counter variable `i` increments by 1.
The result? The program prints:
The sum is: 55
Instead of manually adding each number, the for loop handles it efficiently for you.
Advanced uses of the for loop
You can do a lot more with for loops.
For example, you can create a loop that counts backward:
for (int i = 5; i > 0; i--) {
System.out.println("Countdown: " + i);
}
In this case, the loop starts at 5 and counts down, decreasing `i` by 1 with each iteration.
Countdown: 5
Countdown: 4
Countdown: 3
Countdown: 2
Countdown: 1
A common pitfall: forgetting the update
What happens if you forget to update the counter or make a mistake with the condition?
You end up with an infinite loop! For example:
for (int i = 0; i < 5;) {
System.out.println("I’m stuck here! The value of i is: " + i);
}
In this case, the counter variable `i` never gets updated, so it stays at 0, and the loop keeps running because there’s no end in sight!
An infinite loop is something to avoid as it consumes processing resources endlessly, without any time or memory limits.
So, the next time you need to repeat a task, be sure to avoid accidentally creating an infinite loop.