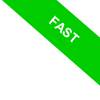
Do-while Loop in Java
The do-while loop in Java is useful when you need to execute a block of code at least once, regardless of whether the condition is initially true or false.
do {
// code block
} while (condition);
Here’s how it works: the code block is executed first, and only after that is the condition checked.
If the condition evaluates to true, the loop runs again. If not, the loop ends.
The key difference between a "do-while" loop and a "while" loop is that in a "do-while" loop, the condition is checked after the code block executes. This ensures that the loop will always run at least once, even if the condition is false. By contrast, in a "while" loop, if the condition is false at the start, the block may never execute at all.
Let’s look at a practical example.
Imagine you need to ask a user to enter a password, and you want to make sure they get at least one chance to input it. If the password is incorrect, you’ll keep asking until they get it right.
import java.util.Scanner;
public class PasswordCheck {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String password;
String correctPassword = "1234";
do {
System.out.print("Enter password: ");
password = scanner.nextLine();
} while (!password.equals(correctPassword));
System.out.println("Correct password! Access granted.");
}
}
In this program, the user is prompted for the password, and the code block inside the "do" will always run at least once.
After the block runs, it checks if the condition !password.equals(correctPassword) is true.
If the condition is true (i.e., the password is incorrect), the loop continues, and the program keeps asking for the password.
Once the correct password is entered, the loop exits and the message "Correct password! Access granted" is displayed.
Example 2
Here’s a simpler example to further illustrate how the "do-while" loop works.
Let’s say you want to print the numbers from 1 to 5:
public class CountToFive {
public static void main(String[] args) {
int number = 10;
do {
System.out.println("Number: " + number);
number++;
} while (number <= 5);
}
}
In this case, the variable 'number' starts at 10. The loop prints the value of 'number' and then increments it (number++).
After each iteration, it checks if the condition number <= 5 is still true.
If 'number' is less than or equal to 5, the loop continues. Otherwise, it terminates.
In this specific example, the output is:
Number: 10
Even though the initial condition was false (since 10 is not less than or equal to 5), the block still executed once, demonstrating that the do-while loop always runs at least once.
When Should You Use the "do-while" Loop?
In general, a "do-while" loop is useful when you need to ensure that a block of code executes at least once, regardless of the condition.
For example, it's ideal for situations where you need to gather user input and want the user to have at least one chance to provide it before any checks are made.
However, the "do-while" loop is not as commonly used as the "while" or "for" loop. In most cases, it's preferable to check the condition before running the block. But, as we’ve seen, there are situations where a do-while loop is the best choice.
I hope this has helped clarify the concept of the do-while loop!
If you have any further questions or would like to see more examples, feel free to reach out!