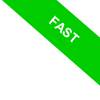
If-Else Conditional Structure in Java
The if-else statement allows you to create a conditional logic structure in your Java programs.
if (condition) {
// Code block X
} else {
// Code block Y
}
The `if` structure enables you to control the flow of your program based on specific conditions.
Simply put, the `if` structure lets you execute a block of code only when a certain condition is met. If the condition is false, that block is skipped. It’s similar to saying, "If X happens, then do Y."
The `else` block is optional and runs only when the initial condition is false.
Think of a situation where you need to make a choice, such as deciding whether to take an umbrella depending on the weather. In programming, the `if` structure is designed for exactly this purpose: making decisions based on specific conditions.
Let's start with a simple example to illustrate how it works.
int temperature = 25;
if (temperature > 20) {
System.out.println("It's warm outside!");
}
This code checks if the temperature is above 20 degrees. If it is, the program prints "It's warm outside!".
If the temperature is 18, for instance, the message won’t be printed because the condition (`temperature > 20`) would be false.
Adding an Alternative with `else`
There are times when you may want something to happen even if the condition is false.
In such cases, you can use the `else` keyword to define an alternative action:
int temperature = 15;
if (temperature > 20) {
System.out.println("It's warm outside!");
} else {
System.out.println("It's cool outside.");
}
Here, if the temperature is above 20, the output will be "It's warm outside!".
Otherwise, in all other cases, the message will be "It's cool outside.".
Handling Multiple Conditions with `else if`
What if you have more than two possibilities? You can use `else if` to handle additional conditions:
int temperature = 10;
if (temperature > 30) {
System.out.println("It's very hot outside!");
} else if (temperature > 20) {
System.out.println("It's warm outside!");
} else if (temperature > 10) {
System.out.println("It's mild outside.");
} else {
System.out.println("It's cold outside.");
}
In this example, the program evaluates each condition in sequence.
The first condition that is true determines which block of code will be executed.
If none of the conditions are met, the final `else` block is executed.
It’s a good practice to include an `else` clause at the end of your conditional structure to handle any situations where none of the previous conditions are true.
A Practical Example
Consider a scenario where you need to write a program that suggests appropriate clothing based on the temperature:
int temperature = 10;
if (temperature > 30) {
System.out.println("It's very hot outside!");
} else if (temperature > 20) {
System.out.println("It's warm outside!");
} else if (temperature > 10) {
System.out.println("It's mild outside.");
} else {
System.out.println("It's cold outside.");
}
If the temperature is 8 degrees, for example, the program might suggest wearing a sweater.
This example demonstrates how the `if` structure can be utilized to make decisions that directly influence the behavior of your program.
In conclusion, the if-else statement is a fundamental conditional structure in Java. As you become more familiar with it, you’ll find it to be an invaluable tool for managing complex decision-making and logic in your programs.