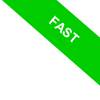
Else if Clause in Java
The `else if` clause allows you to handle multiple conditions in a Java program efficiently.
The basic construct for decision-making in Java is the `if` statement. However, what if you need to evaluate multiple conditions one after another until one is true? This is where the `else if` clause becomes useful.
The `else if` construct is essentially a combination of an `if` statement followed by an `else`.
Here’s how it looks in Java:
if (condition1) {
// code to run if condition1 is true
} else if (condition2) {
// code to run if condition1 is false but condition2 is true
} else if (condition3) {
// code to run if both condition1 and condition2 are false, but condition3 is true
} else {
// code to run if none of the conditions are true
}
This construct allows the program to evaluate multiple conditions in sequence, stopping once it finds one that’s true.
If a condition is met, the corresponding block of code is executed, and any remaining conditions are ignored.
What’s the difference between else and else if?
The `else` and `else if` clauses serve distinct purposes, even though they might seem similar at first glance:
- The `else if` clause checks an additional condition when the initial `if` is false. You can follow it with more `else if` clauses to check other conditions. The program stops checking further conditions as soon as it finds a true `else if`. Unlike a switch-case construct, there’s no need to add a break statement.
- The `else` clause, on the other hand, runs only if all preceding conditions (`if` and `else if`) are false. It doesn’t evaluate any condition itself but serves as a fallback when none of the previous checks are true.
In short, `else if` adds extra conditional checks, while `else` executes only when no earlier conditions are true.
A Practical Example
Let’s walk through a practical example to illustrate this.
Suppose you want to write a program that assigns a letter grade to a numerical score based on the following scale:
- If the score is 90 or higher, it assigns an "A".
- If the score is between 80 and 89, it assigns a "B".
- If the score is between 70 and 79, it assigns a "C".
- If the score is between 60 and 69, it assigns a "D".
- Otherwise, it assigns an "F".
Here’s how you can implement this using `else if`:
public class Grade {
public static void main(String[] args) {
int score = 85; // example score
if (score >= 90) {
System.out.println("Grade is A");
} else if (score >= 80) {
System.out.println("Grade is B");
} else if (score >= 70) {
System.out.println("Grade is C");
} else if (score >= 60) {
System.out.println("Grade is D");
} else {
System.out.println("Grade is F");
}
}
}
Now, let’s break down how this works step by step:
- The program first checks the condition: `score >= 90`.
- If it’s true (for example, if `score` is 92), the program will output `"Grade is A"` and stop evaluating any further conditions.
- If the first condition is false, it moves on to the next one: `score >= 80`. If this is true, the program outputs `"Grade is B"` and stops evaluating the remaining conditions.
- If the second condition is also false, the program continues to check each subsequent `else if` condition, and finally, if none are true, it executes the final `else` block.
In this case, since `score = 85`, the program satisfies the second `else if` condition, and the output is:
Grade is B
Notice that once the program finds a true `else if` condition, it stops evaluating any further conditions.
For example, after determining that `else if (score >= 80)` is true, it ignores the remaining `else if` and `else` clauses, exiting the conditional structure.
Let’s look at another example.
Suppose you want to create a program that gives advice based on the temperature:
int temperature = 25;
if (temperature > 30) {
System.out.println("It's hot, grab a cold drink.");
} else if (temperature >= 20) {
System.out.println("The weather is perfect, enjoy your day.");
} else if (temperature >= 10) {
System.out.println("It's cool, wear a jacket.");
} else {
System.out.println("It's cold, stay warm!");
}
Here, the variable `temperature` is set to 25.
Since this satisfies the second `else if` condition, the program outputs:
The weather is perfect, enjoy your day.
In summary, the `else if` clause allows you to manage multiple conditions efficiently, improving both readability and performance in your code.
Why Use `else if`?
Without the `else if` clause, you’d have to write multiple separate `if` statements, which wouldn’t be as efficient.
When the program finds a true condition in a series of `else if` clauses, it stops checking the remaining conditions, saving time and resources.
On the other hand, if you used separate `if` statements instead, each one would be evaluated independently, even if a previous condition was already met.
Here’s a less efficient example without `else if`:
if (score >= 90) {
System.out.println("Grade is A");
}
if (score >= 80) {
System.out.println("Grade is B");
}
if (score >= 70) {
System.out.println("Grade is C");
}
if (score >= 60) {
System.out.println("Grade is D");
}
if (score < 60) {
System.out.println("Grade is F");
}
In this scenario, even if the score is 85, the program would still evaluate all the remaining conditions after printing `"Grade is B"`, which is unnecessary and inefficient.
Hopefully, this clarifies how `else if` works and why it's a valuable tool for controlling program flow efficiently.