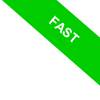
Switch-case Structure in Java
In Java, the switch-case structure is a powerful tool for handling multiple decision paths in your program. It’s particularly useful when you need to execute different actions based on the value of a variable.
Why use it? Imagine you need to make decisions based on the day of the week. While you could use several consecutive if-else statements, Java offers a clearer and more efficient solution: the switch construct. Switch statements are much more readable than a series of nested if-else blocks, making your code easier to understand when managing many alternatives.
Here’s how it works: you pass a variable into the switch statement, and it "chooses" which block of code to execute based on the value of that variable.
switch (variable) {
case value1:
// Code to execute if variable equals value1
break;
case value2:
// Code to execute if variable equals value2
break;
default:
// Code to execute if no value matches
}
In the switch(variable) statement, you provide the variable you want to compare. This can be a number, a string, or an enumeration.
Each case clause represents a potential value for that variable.
If the value matches, the corresponding code block is executed.
It’s essential to include the break statement at the end of each case block. This tells Java to exit the switch statement once the matching case has been executed. Without the break, Java will continue executing the subsequent cases even if they don’t match—this behavior is known as fall-through.
The default: block is executed if none of the cases match the variable’s value.
While the default clause is optional, it’s a good practice to include it to handle unexpected or undefined values, ensuring your program behaves predictably in all scenarios.
Practical Example
Let’s say you want to print the day of the week based on an integer (1 for Monday, 2 for Tuesday, and so on).
int day = 3;
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
case 4:
System.out.println("Thursday");
break;
case 5:
System.out.println("Friday");
break;
case 6:
System.out.println("Saturday");
break;
case 7:
System.out.println("Sunday");
break;
default:
System.out.println("Invalid number");
}
In this example, the variable `day` is 3, so the case 3 block will be executed, printing "Wednesday."
Wednesday
If the variable had a value outside the range of 1-7, the default block would execute, printing "Invalid number."
When Should You Use the Switch Structure?
Switch statements are ideal when you need to evaluate a variable against several possible values.
For just two or three conditions, an if-else might be simpler and more readable.
However, when handling more conditions—like the days of the week—a switch statement keeps your code more organized and easier to follow.
Using Switch with Strings
Java also supports using strings within a switch statement, which can be quite handy.
Here’s an example using the names of the days of the week.
String day = "Monday";
switch (day) {
case "Monday":
System.out.println("Start of the week!");
break;
case "Friday":
System.out.println("Almost the weekend!");
break;
case "Sunday":
System.out.println("Relax, it's Sunday.");
break;
default:
System.out.println("Unknown day");
}
In this case, the variable `day` is a string, and the program prints "Start of the week!" because it matches the case "Monday."
Start of the week!
Make sure not to forget the break statement, as it prevents the execution from continuing through subsequent cases unintentionally.
Fall-through Behavior
Fall-through occurs in a switch when you omit the break statement, causing the program to continue executing subsequent case blocks, even if they don’t match the variable’s value.
In general, fall-through is avoided to prevent unintended behavior, but there are situations where it can be useful.
For example, fall-through lets you group multiple cases together.
int month = 6;
switch (month) {
case 1: case 2: case 3:
System.out.println("It's winter");
; break;
case 4: case 5: case 6:
System.out.println("It's spring");
break;
case 7: case 8: case 9:
System.out.println("It's summer");
break;
case 10: case 11: case 12:
System.out.println("It's autumn");
break;
default:
System.out.println("Invalid month");
}
In this example, the variable 'month' is 6, so the case 4, 5, 6 block is executed, printing "It's spring."
It's spring
Here, the fall-through is intentional: by grouping multiple cases, we can handle several values with a single instruction.
Switch Expressions (Java 12+)
Starting with Java 12, the switch statement was enhanced with switch expressions.
For example, you can now assign a value directly to a variable without needing the break statement:
String season = switch (month) {
case 12, 1, 2 -> "Winter";
case 3, 4, 5 -> "Spring";
case 6, 7, 8 -> "Summer";
case 9, 10, 11 -> "Autumn";
default -> "Invalid month";
};
System.out.println(season);
This syntax makes the code even more concise and easier to read.
Keep in mind that switch expressions are only available in recent versions of Java!