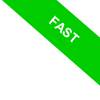
While Loop in Java
The `while` loop is a fundamental tool in Java that allows you to create a conditional loop. It's commonly used to repeat an action a set number of times or until a certain condition is met.
while (condition) {
// code block
}
The code block can consist of one or multiple lines.
How does it work?
The `while` loop keeps executing as long as the specified condition remains true.
Each time the `while` loop runs, it completes one iteration. So, a loop can consist of multiple iterations, where each iteration represents a single "pass" through the loop.
In other words, before starting a new iteration, the `while` loop checks if the condition is still true. It's like asking, "Can I continue?" If the answer is yes, the loop executes the code block and asks the same question again. If the answer is no, the loop stops, and the program moves on.
A Simple Example
Here’s a straightforward example of a `while` loop.
int count = 0;
while (count < 5) {
System.out.println("The value of count is: " + count);
count++; // increments count by 1
}
This code starts by setting the `count` variable to zero.
The loop condition is `count < 5`, so the loop will continue to execute as long as the value of `count` is less than 5.
Each time the loop runs, it prints the current value of `count` and increments it by 1 (`count++` means "add 1 to `count`").
Once `count` reaches 5, the condition `count < 5` is no longer true, and the loop stops.
The output of this program will be:
The value of count is: 0
The value of count is: 1
The value of count is: 2
The value of count is: 3
The value of count is: 4
What if the condition is false from the start?
If the condition is false when the loop starts, the `while` loop won’t execute at all, not even once.
For example, look at this code:
int count = 10;
while (count < 5) {
System.out.println("You’ll never see this message");
}
In this case, since `count` is already greater than 5, the loop never begins.
If you want to ensure that a loop runs at least once, consider using the do-while loop.
Watch out for infinite loops! If you don’t update the variable in the condition, the loop might run endlessly, creating an infinite loop.
int count = 0;
while (count < 5) {
System.out.println("This loop will run forever...");
// oops, we forgot to increment count!
}
In this case, `count` remains 0, so the condition `count < 5` is always true. The loop will never stop running, potentially freezing your program!
Another Example
Let’s say you want to keep adding numbers until the total sum exceeds 100. Here’s how you could do it:
int sum = 0;
int number = 1;
while (sum <= 100) {
sum += number;
System.out.println("Added " + number + ", current sum: " + sum);
number++;
}
In this example, the sum continues to increase until it exceeds 100.
After each addition, the program prints the current sum.
Once the sum reaches or surpasses 100, the loop stops.
Added 1, current sum: 1
Added 2, current sum: 3
Added 3, current sum: 6
Added 4, current sum: 10
Added 5, current sum: 15
Added 6, current sum: 21
Added 7, current sum: 28
Added 8, current sum: 36
Added 9, current sum: 45
Added 10, current sum: 55
Added 11, current sum: 66
Added 12, current sum: 78
Added 13, current sum: 91
Added 14, current sum: 105
Differences Between the `while` and `for` Loops
The `while` loop is typically used when you don’t know in advance how many times you need to repeat the code block.
These loops are often referred to as indeterminate loops.
In an indeterminate loop, the end is based on a condition that may change unpredictably. As a result, the number of iterations the loop will perform is unknown at the start.
The `for` loop, on the other hand, is used when you know in advance how many iterations the loop will perform. Thus, a `for` loop isn’t suited for indeterminate loops.
To handle indeterminate loops, you can only use a `while` or `do-while` loop.
Determinate Loops
A loop is called "determinate" when the number of iterations is known beforehand.
If you know exactly how many times the loop needs to run, you can choose between a `for` loop:
for (int i = 0; i < 5; i++) {
System.out.println("The value of count is: " + i);
}
or a `while` loop:
int count = 0;
while (count < 5) {
System.out.println("The value of count is: " + count);
count++; // increment count by 1
}
In both cases, the output will be the same:
The value of count is: 0
The value of count is: 1
The value of count is: 2
The value of count is: 3
The value of count is: 4
In conclusion, the `while` loop allows you to create both determinate and indeterminate loops. It offers more flexibility.
The `for` loop, however, is only suitable for determinate loops, where you know from the start how many iterations will be needed.
When using a `while` loop, always be cautious of infinite loops, and ensure that there's a way to "exit" the loop by modifying the control variable. Here’s a useful tip: every time you write a loop, ask yourself, "When and how will this loop end?" Always ensure there’s a clear exit strategy. For example, you can force the loop to end after a certain number of iterations, regardless of the condition.
I hope this explanation was helpful! If you have any questions or would like to see more examples, feel free to ask in the comments below.