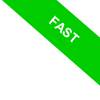
Increment and Decrement Operators in Java
In Java, the increment (`++`) and decrement (`--`) operators are used to modify the value of a numeric variable by increasing or decreasing it by 1.
Operator | Description | Example |
---|---|---|
++ | Increment | a++ or ++a |
-- | Decrement | a-- or --a |
These are some of the first tools you learn when starting out in programming.
They can be used in various scenarios, such as within loops or when you simply need to update the value of a counter.
How do they work?
Here’s a breakdown of how these operators function:
- `++`: Increases the variable's value by 1.
- `--`: Decreases the variable's value by 1.
For example, to increment the value of the variable x
, you could write x = x + 1.
int x = 10;
x = x + 1;
System.out.println(x);
11
Alternatively, you can use the increment operator, x++.
int x = 10;
x++;
System.out.println(x);
11
The end result is the same.
On the other hand, using the decrement operator decreases the variable's value by one.
int x = 10;
x--;
System.out.println(x);
9
While these operators might seem straightforward, there are a few nuances to consider that make them worth exploring.
In particular, it's important to understand the difference between using these operators in prefix form (before the variable) and postfix form (after the variable).
Prefix vs. Postfix Operators
The increment (`++`) and decrement (`--`) operators can be used in two ways:
- Prefix (`++x`): Increments the variable's value before it is used in an expression.
- Postfix (`x++`): Increments the variable's value after the expression has been evaluated.
These two forms behave slightly differently, especially in more complex expressions.
Let's look at a practical example to clarify this.
int x = 5;
int y = ++x;
System.out.println(y);
System.out.println(x);
Here, the prefix operator (`++x`) increments the variable x
to 6 before its value is assigned to y
.
So, the final value of y
will be 6.
6
6
Now, let’s use the postfix operator instead.
int x = 5;
int y = x++;
System.out.println(y);
System.out.println(x);
In this case (`x++`), the current value of x
(which is 5) is assigned to y
first, and only then is x
incremented to 6.
Therefore, the final value of y
is 5.
5
6
Here’s another example, this time with the decrement operator:
int x = 10;
int y = --x;
System.out.println(y);
System.out.println(x);
In this instance, the prefix decrement operator `--` reduces the value of x
before the assignment occurs.
9
9
Now, let’s use the postfix operator.
int x = 10;
int y = x--;
System.out.println(y);
System.out.println(x);
Here, x
is decremented only after the assignment.
10
9
When to Use Prefix and Postfix Operators?
In most cases, especially in loops like a for
loop, the postfix operator is commonly used because the exact timing of the increment or decrement doesn’t impact the outcome:
for (int i = 0; i < 5; i++) {
System.out.println(i);
}
In this loop, `i++` means "increment i
by 1 after evaluating the loop condition and executing the loop body."
However, using `++i` here would yield the same behavior, so either is fine in this context.
When the operator is part of a more complex expression, such as when assigning a value to another variable, the choice between prefix and postfix becomes crucial, as we saw in the examples.
For instance, in this code, the `++` operator is postfix, so the variable x
is multiplied by two first and then incremented:
int x = 5;
int y = (x++) * 2;
System.out.println(y);
10
In contrast, when using the prefix form, the variable x
is incremented first and then multiplied by two:
int x = 5;
int y = (++x) * 2;
System.out.println(y);
12
Therefore, it’s important to understand how these operators modify a variable's value to avoid unexpected results!
Before using them, make sure you're clear on the differences between prefix and postfix forms to prevent unintended behavior in your code.