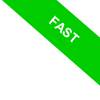
Java Cast Operator
Cast operators in Java allow you to force one data type to be treated as another.
What is casting? Casting lets you take a piece of data of one type and convert it into another. However, this isn't always safe, and Java will alert you if you're attempting to force a conversion that doesn't make sense.
In Java, there are two main types of casting:
- Implicit casting (automatic): when Java can perform the conversion for you without any intervention.
- Explicit casting (manual): when you need to specifically instruct Java to perform the conversion, even if it has concerns.
Java performs implicit casting when it’s confident that no data will be lost. However, when there’s a risk of losing information, explicit casting is required.
In addition to primitive types (such as `int`, `double`, `float`, etc.), casting can also be applied to objects. This adds a layer of complexity and interest.
Let’s walk through three examples to illustrate how casting works in different contexts.
Example of implicit casting
If you have an `int` variable (which occupies less memory) and you want to assign it to a `double` variable (which takes up more space), Java will handle this seamlessly:
int number = 5;
double largerNumber = number; // This is implicit casting.
System.out.println(largerNumber);
Output: 5.0
Why does this work? Because a `double` can easily accommodate an `int`.
It’s like putting a small object in a large drawer — Java doesn't mind, it handles this gladly!
Example of explicit casting
Now, let’s try the reverse: you have a `double` and want to assign it to an `int`.
Here, things are different because a `double` can store decimal values, while an `int` cannot.
You need to explicitly tell Java: "Yes, I know this could be risky, but I’m going ahead with it!"
double largeNumber = 5.75;
int smallNumber = (int) largeNumber; // This is explicit casting.
System.out.println(smallNumber);
5
What happened? The value 5.75 was “truncated”—the decimal part was simply dropped.
Java doesn’t round numbers; it’s strict: it only keeps the integer portion. So, 5.75 becomes 5.
Casting with objects
Imagine you have a class `Animal` and a subclass `Dog`.
Since a dog is an animal, an object of type `Dog` can be treated as an object of type `Animal`. This demonstrates Java's polymorphism capabilities.
However, if you have an object of type `Animal`, you can’t just treat it as a `Dog` unless it actually is a dog!
Here’s a practical example.
class Animal {
public void makeSound() {
System.out.println("An animal makes a sound...");
}
}
class Dog extends Animal {
public void makeSound() {
System.out.println("The dog barks!");
}
public void wagTail() {
System.out.println("The dog wags its tail.");
}
}
public class Main {
public static void main(String[] args) {
Animal myAnimal = new Dog(); // A dog is an animal.
myAnimal.makeSound(); // Prints: "The dog barks!"
// Explicit casting
Dog myDog = (Dog) myAnimal; // Now myAnimal is treated as a Dog.
myDog.wagTail(); // Prints: "The dog wags its tail."
}
}
In this example, the dog is initially treated as an `Animal`, and then, with explicit casting, as a full-fledged `Dog`, complete with all its features (like tail-wagging).
The dog barks!
The dog wags its tail.
However, if you attempt to cast an object that isn't actually of the type you're trying to cast to, Java will throw a runtime error.
Animal myAnimal = new Animal();
Dog myDog = (Dog) myAnimal;
Exception in thread "main" java.lang.ClassCastException: class Animal cannot be cast to class Dog
Why doesn’t this work? Because you're trying to make a generic animal behave like a dog, but that specific animal doesn’t have dog-like characteristics. It hasn't learned how to wag its tail!
In conclusion, cast operators in Java allow you to "force" one data type to behave as another, whether you're dealing with primitive types or objects.