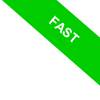
Logical Operators in Java
In Java, logical operators are used to combine boolean expressions—those that evaluate to either true or false. Below is an overview of the primary logical operators:
Operator | Description | Example |
---|---|---|
&& | Logical AND | a && b |
|| | Logical OR | a || b |
! | Logical NOT | !a |
With these operators, you can handle virtually any logical scenario in your program.
Let's explore how each one operates in Java.
Why use them? Imagine a world made up entirely of "true" and "false." This is the reality in which computers function: a binary environment where every decision comes down to two simple values. In Java, as in most programming languages, we use these values—true and false—to make decisions.
The `&&` Operator (Logical AND)
The `&&` (Logical AND) operator checks two conditions and returns true only if both are true.
Both conditions must be true for the entire expression to be evaluated as true.
For example, imagine you want to go for a walk, but you'll only go if:
- The weather is nice
- You have enough free time
If both conditions are true, you'll go out. In Java, this can be represented as follows:
boolean isSunny = true;
boolean hasFreeTime = true;
if (isSunny && hasFreeTime) {
System.out.println("Let's go for a walk!");
} else {
System.out.println("We can't go for a walk.");
}
The `&&` operator checks if both `isSunny` and `hasFreeTime` are true.
In this case, since both are true, the program will output:
Let's go for a walk!
If even one of them is false, the result will be false, and the message will read "We can't go for a walk."
The `||` Operator (Logical OR)
The `||` (Logical OR) operator checks two conditions and returns true if at least one of them is true. This makes it more flexible than the AND operator.
Let's revisit the walking example. Now, you’re willing to go for a walk if:
- The weather is nice OR
- You feel like walking (even if the weather isn't great)
Here’s how you might express this in Java:
boolean isSunny = false;
boolean wantsToWalk = true;
if (isSunny || wantsToWalk) {
System.out.println("Let's go for a walk!");
} else {
System.out.println("Let's stay home.");
}
The `||` operator checks if at least one of the conditions (`isSunny` or `wantsToWalk`) is true.
Since `wantsToWalk` is true, the overall result is true, and it prints:
Let's go for a walk!
If both conditions are false, the code will output "Let's stay home."
The `!` Operator (Logical NOT)
The `!` (Logical NOT) operator reverses the value of a condition. In other words, it inverts the result: if it's true, it becomes false, and vice versa.
Imagine you only want to go out if it's not raining. Here's how you could represent this in Java:
boolean isRaining = false;
if (!isRaining) {
System.out.println("Let's go for a walk!");
} else {
System.out.println("Better stay inside.");
}
The condition `!isRaining` effectively means "it's not raining."
Here, since `!isRaining` is true, the program outputs:
Let's go for a walk!
Combining Logical Operators
Logical operators can be combined to create more complex conditions.
Suppose you want to go out only if:
- The weather is nice AND you have free time, OR the weather is bad, but you still want to go out
In Java, this can be expressed as follows:
boolean isSunny = true;
boolean hasFreeTime = false;
boolean wantsToWalk = true;
if (((isSunny && hasFreeTime) || (!isSunny && wantsToWalk)) {
System.out.println("Let's go for a walk!");
} else {
System.out.println("Better stay home.");
}
Here, we combined logical operators to account for multiple scenarios.
Parentheses are crucial, just like in mathematics, as they define the order in which conditions are evaluated.
Be Mindful of Short-Circuiting!
In Java, the `&&` and `||` operators perform "short-circuiting."
This means that the evaluation stops as soon as the outcome is determined.
For example, with the `&&` operator, if the first condition is false, Java won't even evaluate the second one because the entire expression will be false. Similarly, with `||`, if the first condition is true, Java ignores the second.
Here's an example:
boolean isSunny = false;
boolean hasFreeTime = true;
if (isSunny && (hasFreeTime = false)) {
System.out.println("Let's go for a walk!");
}
System.out.println("Free time: " + hasFreeTime);
Even though the code assigns `false` to `hasFreeTime` in the second condition, it is never evaluated because the first condition (`isSunny`) is already false.
As a result, `hasFreeTime` remains true, illustrating the short-circuiting behavior of the `&&` operator.