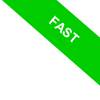
Relational Operators in Java
Relational operators in Java are used to compare two values, returning a boolean result: either `true` or `false`, depending on the outcome of the comparison.
The key relational operators in Java are:
Operator | Description | Example |
---|---|---|
== | Equal to | a == b |
!= | Not equal to | a != b |
> | Greater than | a > b |
< | Less than | a < b |
>= | Greater than or equal to | a >= b |
<= | Less than or equal to | a <= b |
Relational operators are essential when making decisions in your programs, especially when using conditional structures. They allow you to control the flow of your code by comparing values based on the conditions you define.
Let’s explore each of these operators with practical examples to see how they work.
The Equal To Operator (==)
Imagine you’re in a store and want to decide whether to buy an item based on its price.
You have a fixed budget and need to check if the item costs exactly what you're willing to spend. You might write:
int itemPrice = 50;
int budget = 50;
if (itemPrice == budget) {
System.out.println("The item costs exactly what you can afford!");
}
The item costs exactly what you can afford!
In this case, the `==` operator compares `itemPrice` and `budget`.
If they are equal, the condition is true, and the message "The item costs exactly what you can afford!" will be printed.
The Not Equal To Operator (!=)
If you want to buy an item only if it doesn't cost exactly 50 euros, you can use the `!=` operator, which means "not equal to":
int itemPrice = 50;
int budget = 20;
if (itemPrice != budget) {
System.out.println("The item's price is different from what you set.");
}
The item's price is different from what you set.
Here, `!=` checks if `itemPrice` is not equal to `budget`. If this is true, the condition is satisfied.
The Greater Than Operator (>)
Let's say you want to check if an item is too expensive for you:
int itemPrice = 50;
int budget = 20;
if (itemPrice > budget) {
System.out.println("The item is too expensive!");
}
The item is too expensive!
The `>` operator checks if `itemPrice` is greater than `budget`. If it is, the condition is true.
The Less Than Operator (<)
Similarly, you can check if the item's price is below your budget:
int itemPrice = 50;
int budget = 100;
if (itemPrice < budget) {
System.out.println("You can afford the item!");
}
You can afford the item!
The `<` operator verifies if `itemPrice` is less than `budget`. If so, the condition is satisfied.
The `>=` and `<=` Operators
These operators are combinations: they check if a value is either greater than or equal to (`>=`) or less than or equal to (`<=`) another. They’re useful when you want to include the case where the values are equal.
For example, if you want to buy the item only if its price is within or equal to your budget:
int itemPrice = 50;
int budget = 50;
if (itemPrice <= budget) {
System.out.println("The item is within or exactly at your budget limit.");
}
The item is within or exactly at your budget limit.
In this scenario, since the two values are equal, the condition is met.
Be cautious when comparing objects. Relational operators like `==` and `!=` work well with primitive types (such as `int`, `double`, etc.), but they don't behave the same way when used with objects.
When comparing objects in Java, using `==` isn't the best approach, as it checks if the objects reference the same memory location, not if their contents are the same.
Example 1
Consider comparing two variables that point to the same string 'Tom'.
String name1 = "Tom";
String name2 = "Tom";
if (name1==name2) {
System.out.println("The names are the same!");
} else {
System.out.println("The names are NOT the same!");
}
The names are the same!
In this case, you're dealing with string literals.
In Java, string literals are managed through the String Pool, a special memory area where Java stores strings. To optimize memory usage, if two variables have the same string literal, they point to the same memory address.
When you create a string literal, Java checks if an identical string already exists in the pool. If it does, it reuses the existing reference instead of creating a new one.
Thus, the comparison returns true because both variables reference the same memory address.
Example 2
Now, let's create two distinct objects that contain the same string value:
String name1 = new String("Tom");
String name2 = new String("Tom");
if (name1==name2) {
System.out.println("The names are the same!");
} else {
System.out.println("The names are NOT the same!");
}
The names are NOT the same!
Here, the two objects have different memory references since they occupy separate locations in memory.
As a result, Java indicates that they are not the same.
Example 3
If you want to compare the content of two strings when working with objects, use the `.equals()` method:
String name1 = new String("Tom");
String name2 = new String("Tom");
if (name1.equals(name2)) {
System.out.println("The names are the same!");
} else {
System.out.println("The names are NOT the same!");
}
The names are the same!