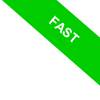
The operations of vector calculus in Octave
In this lesson I'll explain how to do the main operations of vector calculus in Octave with some practical examples.
Define a column vector in 3-dimensional space.
>> v=[1; 3; 4;]
Define another column vector in the same space
>> w=[2; 1; -1]
Here are some mathematical operations between the two vectors
Sum of two vectors
To add the two vectors type v+w
>> v+w
ans =
3
4
3
Explication. $$ \vec{v} + \vec{w} = \begin{pmatrix} 1 \\ 3 \\ 4 \end{pmatrix} + \begin{pmatrix} 2 \\ 1 \\ -1 \end{pmatrix} = \begin{pmatrix} 1+2 \\ 3+1 \\ 4-1 \end{pmatrix} = \begin{pmatrix} 3 \\ 4 \\ 3 \end{pmatrix} $$
Difference between two vectors
To subtract the two vectors, type v-w
>> v-w
ans =
-1
2
5
Explication. $$ \vec{v} - \vec{w} = \begin{pmatrix} 1 \\ 3 \\ 4 \end{pmatrix} - \begin{pmatrix} 2 \\ 1 \\ -1 \end{pmatrix} = \begin{pmatrix} 1-2 \\ 3-1 \\ 4-(-1) \end{pmatrix} = \begin{pmatrix} -1 \\ 2 \\ 5 \end{pmatrix} $$
Multiplication between two vectors
Per moltiplicare due vettori colonna devi trasformare uno dei due vettori in un vettore riga tramite una trasposizione.
To transpose a vector in Octave you just need to add a superscript to the right of the array variable name.
>> v*w'
ans =
2 1 -1
6 3 -3
8 4 -4
Explication. $$ \vec{v} \cdot \vec{w}^T = \begin{pmatrix} 1 \\ 3 \\ 4 \end{pmatrix} \cdot \begin{pmatrix} 2 & 1 & -1 \end{pmatrix} = \begin{pmatrix}1 \cdot 2 & 1 \cdot 1 & 1 \cdot (-1) \\3 \cdot 2 & 3 \cdot 1 & 3 \cdot (-1) \\ 4 \cdot 2 & 4 \cdot 1 & 4 \cdot (-1) \end{pmatrix} = \begin{pmatrix}2 & 1 & -1 \\ 6 & 3 & -3 \\ 8 & 4 & -4 \end{pmatrix} $$
Multiplication between vectors does not respect the commutative property. Therefore, multiplying v '* w returns a different result than v * w'
>> v'*w
ans = 1
Explication. $$ \vec{v}^T \cdot \vec{w} = \begin{pmatrix} 1 & 3 & 4 \end{pmatrix} \cdot \begin{pmatrix} 2 \\ 1 \\ -1 \end{pmatrix} = \begin{pmatrix} 1 \cdot 2 + 3 \cdot 1 + 4 \cdot (-1) \end{pmatrix} = \begin{pmatrix} 2 + 3 - 4 \end{pmatrix} \begin{pmatrix} 1 \end{pmatrix} = 1 $$
Multiplication of the vector element by element
This is another type of vector multiplication. Element-by-element multiplication calculates the product of the elements of vectors in the same position.
To do this type of multiplication you have to use the operator symbol .*
>> v*w
ans =
2
3
-4
In element-by-element multiplication, the two vectors must both be row vectors (or both column vectors) and have the same size
Explication. $$ \vec{v} \cdot \vec{w} = \begin{pmatrix} 1 \\ 3 \\ 4 \end{pmatrix} \ .* \ \begin{pmatrix} 2 \\ 1 \\ -1 \\ \end{pmatrix} = \begin{pmatrix} 1 \cdot 2 \\ 3 \cdot 1 \\ 4 \cdot -1 \end{pmatrix} = \begin{pmatrix} 2 \\ 3 \\ -4 \end{pmatrix} $$
Multiplication of the vector by a scalar
To multiply a vector by a scalar number, for example k = 2, just write 2*v
>> 2*v
ans =
2
6
8
Explication. $$ 2 \cdot \begin{pmatrix} 1 \\ 3 \\ 4 \end{pmatrix} = \begin{pmatrix} 2 \cdot 1 \\ 2 \cdot 3 \\ 2 \cdot 4 \end{pmatrix} = \begin{pmatrix} 2 \\ 6 \\ 8 \end{pmatrix} $$
Division of the vector by a scalar
Similarly you can divide the vector by a scalar
>> v/2
ans =
0.5
1.5
2.0
Explication. $$ \frac{ \vec{v} }{2} = \begin{pmatrix} 1 \\ 3 \\ 4 \end{pmatrix} \cdot \frac{1}{2} = \begin{pmatrix} \frac{1}{2} \\ \frac{3}{2} \\ \frac{4}{2} \end{pmatrix} = \begin{pmatrix} 0.5 \\ 1.5 \\ 2 \end{pmatrix} $$
In these examples I have used column vectors but the indications are valid even if you use row vectors.
Division of the vector element by element
It is another type of division between two vectors. Element-by-element division calculates the quotient of the elements of the vectors in the same position.
To do this type of division you have to use the operator symbol ./
>> v./v
ans =
0.5
3
-4
In the element-by-element division, the two vectors must be both row vectors (or both column vectors) and have the same size.
Explication. $$ \vec{v} \cdot \vec{w} = \begin{pmatrix} 1 \\ 3 \\ 4 \end{pmatrix} \ ./ \ \begin{pmatrix} 2 \\ 1 \\ -1 \end{pmatrix} = \begin{pmatrix} \frac{1}{2} \\ \frac{3}{1} \\ \frac{4}{-1} \end{pmatrix} = \begin{pmatrix} 0.5 \\ 3 \\ -4 \end{pmatrix} $$
Exponentiation of the vector element by element
Exponentiation element by element raises the elements of the vector by the same exponent.
To do this type of operation you have to use the operator symbol .^
>> v.^2
ans =
1
9
16
In element-by-element exponentiation, the two vectors must both be row vectors (or both column vectors) and have the same size.
Explication. $$ \vec{v} \ \text{.^} \ 2 = \begin{pmatrix} 1^2 \\ 3^2 \\ 4^2 \end{pmatrix} = \begin{pmatrix} 1 \\ 9 \\ 16 \end{pmatrix} $$