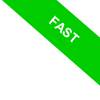
How to make a random matrix in octave
In this lesson I'll explain how to create a matrix with random values in Octave.
I'll give you a practical example
To create a 2x3 matrix randomly use the rand(rows,columns) function
>> rand(2,3)
Octave generates a random matrix with values between 0 and 1
ans =
0.495435 0.651593 0.093860
0.449491 0.788723 0.028347
The numbers generated are different each time and distributed evenly in the interval (0,1).
To create a random array with values between 0 and 10, multiply the rand () function by 10.
>> rand(2,3)*10
ans =
1.35346 1.04275 0.73193
5.51170 0.39138 8.66168
If you want to create a random array with integers, combine the rand() function with the round() function
For example, create a 2x3 matrix with random values between 0 and 10.
>> round(rand(2,3)*10)
ans =
3 9 10
3 6 9
Alternatively, you can type randi(max value, rows, columns) to get the same result.
>> randi(10,2,3)
ans =
1 9 3
2 1 10
Also in this case Octave produces in output a random matrix with integer random values.
How does Octave generate random values? Most of the algorithms that generate random numbers use very long lists with different types of distributions. Hence, they are not true random numbers but pseudorandom values. Octave initializes the algorithm based on the time of the computer clock. Therefore the numbers are always different. However, Octave also allows you to always use the same sequence of random numbers.