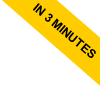
Matrix operations in Octave
In this lesson I'll explain how to do the main matrix operations in Octave with some practical examples.
Before starting, create two matrix arrays.
Write a matrix M1 with two rows and two columns. It's a square matrix.
>> M1=[1 4;2 3]
M 1 =
1 4
2 3
Now write another square matrix M2 with two rows and two columns.
>> M2=[3 1;7 5]
M2 =
3 1
7 5
Here are some operations of the matrix calculus
- Matrix addition
- Matrix subtraction
- Matrix multiplication
- Multiplication of matrices element by element
- Scalar multiplication of matrices
- Matrix division
- Matrix division element by element
- Matrix division by a scalar
- Matrix exponentiation element by element
- Matrix determinant
- Rank
- Transpose matrix
- Inverse matrix
Matrix addition
To add two matrices type M1 + M2
>> M1+M2
ans =
4 5
9 8
Note. $$ M1 + M2 = \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} + \begin{pmatrix} 3 & 1 \\ 7 & 5 \end{pmatrix} = \begin{pmatrix} 1+3 & 4+1 \\ 2+7 & 3+5 \end{pmatrix} = \begin{pmatrix} 4 & 5 \\ 9 & 8 \end{pmatrix} $$
Matrix subtraction
To calculate the difference between two matrices, type M1-M2
>> M1-M2
ans =
-2 3
-5 -2
Note. $$ M1 - M2 = \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} - \begin{pmatrix} 3 & 1 \\ 7 & 5 \end{pmatrix} = \begin{pmatrix} 1-3 & 4-1 \\ 2-7 & 3-5 \end{pmatrix} = \begin{pmatrix} -2 & 3 \\ -5 & -2 \end{pmatrix} $$
Matrix multiplication
To calculate the product between two matrices, type M1 * M2
>> M1*M2
ans =
31 21
27 17
Note. $$ M1 \cdot M2 = \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} \cdot \begin{pmatrix} 3 & 1 \\ 7 & 5 \end{pmatrix} = \begin{pmatrix} 1 \cdot 3 + 4 \cdot 7 & 1 \cdot 1 + 4 \cdot 5 \\ 2 \cdot 3 + 3 \cdot 7 & 2 \cdot 1 + 3 \cdot 5 \end{pmatrix} = \begin{pmatrix} 31 & 21 \\ 27 & 17 \end{pmatrix} $$
Ricorda che puoi calcolare il prodotto due tra matrici solo se il numero di colonne della prima matrice è uguale al numero di righe della seconda matrice
Multiplication of matrices element by element
It is another type of matrix multiplication. This operation calculates the product of the elements of the matrix arrays that occupy the same position.
To calculate this type of multiplication you have to use the symbol .*
>> M1 .* M2
ans =
3 4
14 15
In element-by-element multiplication, the two matrices must have the same number of rows and columns.
Note. $$ M1 \ .* \ M2 = \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} \ .* \ \begin{pmatrix} 3 & 1 \\ 7 & 5 \end{pmatrix} = \begin{pmatrix} 1 \cdot 3 & 4 \cdot 1 \\ 2 \cdot 7 & 3 \cdot 5 \end{pmatrix} = \begin{pmatrix} 3 & 4 \\ 14 & 15 \end{pmatrix} $$
Scalar multiplication of matrices
To multiply a matrix by a scalar number, for example k = 2, type 2 * M1
>> 2*M1
ans =
2 8
4 6
All elements of the matrix are multiplied by the scalar number.
Note. $$ 2 \cdot M1 = 2 \cdot \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} = \begin{pmatrix} 2 \cdot 1 & 2 \cdot 4 \\2 \cdot 2 & 2 \cdot 3 \end{pmatrix} = \begin{pmatrix} 2 & 8 \\4 & 6 \end{pmatrix} $$
Matrix division
In linear algebra the division between two matrices is calculated by multiplying the first matrix by the inverse matrix of the second M1·M2-1.
To calculate this operation in Octave type M1*inv(M2)
>> M1*inv(M2)
ans =
-2.87500 1.37500
-1.37500 0.87500
Alternatively you can also type M1/M2
>> M1/M2
ans =
-2.87500 1.37500
-1.37500 0.87500
The result is the same.
Matrix division element by element
It is another type of matrix division. This operation calculates the quotient between the elements of the arrays that are in the same position.
To do this type of division you have to use the symbol ./
>> M1 ./ M2
ans =
0.33333 4.0000
0.28571 0.6000
In the element-by-element division, the two matrices must have the same number of rows and columns.
Note. $$ M1 \ ./ \ M2 = \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} \ ./ \ \begin{pmatrix} 3 & 1 \\ 7 & 5 \end{pmatrix} = \begin{pmatrix} \frac{1}{3} & \frac{4}{1} \\ \frac{2}{7} & \frac{3}{5} \end{pmatrix} = \begin{pmatrix} 0.33333 & 0.28571 \\ 4 & 0.6 \end{pmatrix} $$
Matrix division by a scalar
If you want to divide a matrix by a scalar number, for example k = 2, type M1 / 2
>> M1/2
ans =
0.50000 2.00000
1.00000 1.50000
The elements of the matrix are divided by the scalar number.
Note. $$ \frac{M1}{2} = \frac{1}{2} \cdot \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} = \begin{pmatrix} \frac{1}{2} \cdot 1 & \frac{1}{2} \cdot 4 \\ \frac{1}{2} \cdot 2 & \frac{1}{2} \cdot 3 \end{pmatrix} = \begin{pmatrix} 0.5 & 2 \\ 1 & 1.5 \end{pmatrix} $$
Matrix exponentiation element by element
This operation calculates the exponentiation of all the elements of the matrix by the same exponent.
To do this type of operation you have to use the symbol .^
>> M1.^2
ans =
1 16
4 9
Note. $$ M1 \ \text{.^} \ 2 = \begin{pmatrix} 1^2 & 4^2 \\ 2^2 & 3^2 \end{pmatrix} = \begin{pmatrix} 1 & 16 \\ 4 & 9 \end{pmatrix} $$
Matrix determinant
To calculate the determinant of a square matrix use the det() function
>> det(M1)
ans = -5
Note. $$ \text{det} (M1) = det \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} = 1 \cdot 3 - 4 \cdot 2 = -5 $$
Rank
To calculate the rank of a square matrix use the rank() function
>> rank(M1)
ans = 2
Transpose matrix
To transpose a matrix use the transpose() function
>> transpose(M1)
ans =
1 2
4 3
Alternatively, you can transpose the matrix by adding a single quote after the matrix name
>> M1'
ans =
1 2
4 3
Note. In the transposition, the rows of the matrix become columns. $$ \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix}^T = \begin{pmatrix} 1 & 2 \\ 4 & 3 \end{pmatrix} $$
Inverse matrix
To calculate the inverse matrix use the function inv()
>> inv(M1)
ans =
-0.60000 0.80000
0.40000 -0.20000
Note. The inverse matrix of M1 is a matrix that multiplied by M1 results in an identity matrix, i.e. a matrix with elements equal to 1 on the main diagonal and all other elements are null. $$ M1 \cdot \text{inv} (M1) = \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} \cdot \begin{pmatrix} -0.6 & 0.8 \\ 0.4 & -0.2 \end{pmatrix} = \begin{pmatrix} 1 & 0 \\ 0 & 1 \end{pmatrix} $$