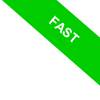
Hybrid Modules in Python
In the Python ecosystem, "hybrid modules" refer to a versatile file format that can double as both executable scripts and a repository for classes and functions.
Creating a Hybrid Module The key to forging a hybrid module lies in the strategic use of a conditional block. This block discerns whether the file is being run directly or imported, by inspecting the module's "__name__" attribute.
The "__name__" attribute behaves differently based on the file's usage:
- If executed directly as a script, this attribute automatically takes on the value "__main__".
- However, when imported as a module, its value mirrors the name of the module file, sans the ".py" extension.
This mechanism allows for selective code execution, enabling you to craft code with dual behavior based on its execution context.
Case Study
Consider a module capable of calculating a number's factorial among its functionalities.
Our goal is for the factorial calculation to proceed when the module operates as a script. However, we aim to prevent automatic execution upon the module's importation into another script.
def calculate_factorial(n):
"""Calculates the factorial of a given number n."""
if n < 0:
return None
result = 1
for i in range(1, n + 1):
result *= i
return result
def main():
number = int(input("Enter a number to calculate its factorial: "))
result = calculate_factorial(number)
print(f"The factorial of {number} is {result}")
if __name__ == "__main__":
main()
Store this code in a file named "factorial.py".
Here's how the module's behavior adapts under different circumstances:
1] Script Execution
Running this file as a script sets the module's __name__ attribute to "__main__". The code identifies this condition with a conditional block, triggering the main() function automatically.
This prompts the user to input a number, leading to the calculation and display of the number's factorial.
Enter a number to calculate its factorial:
2] Module Importation
Importing the file with the import statement, while omitting the ".py" extension, sets the __name__ attribute to the module's name.
The absence of "__main__" in the conditional block prevents the main() function from executing.
Thus, the functions are loaded without running the module, bypassing any user prompts for number input.
Post-import, you can invoke the calculate_factorial() function either from the command line or within another script.
>>> import factorial
>>> factorial.calculate_factorial(5)
120
This exemplifies the essence of a hybrid module: its functionality diverges based on usage, marrying the roles of a reusable module and a standalone script.
Such hybrid modules shine by offering the flexibility to serve both as modular, reusable components and as independent applications. This not only fosters code reuse and ease of maintenance but also enhances efficiency and organization within your Python projects, streamlining their structure for better modularity and maintainability.