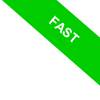
Python's random Module
Let's dive into Python's random module, an in-built library that allows you to generate random numbers.
This module is incredibly versatile, suitable for a variety of scenarios including simulations, gaming, algorithm testing, and even cryptography. For cryptographic purposes, however, the `secrets` module is a more secure alternative.
Starting with the basics, the random module allows you to generate a random number with ease.
import random
# Generate a random integer between 1 and 10
number = random.randint(1, 10)
print(number)
This snippet churns out a random integer between 1 and 10 using the randint() method, illustrating the simplicity and power of the module right from the get-go.
For instance, consider the number 3:
3
This is merely scratching the surface, as the random module conceals a wealth of functionalities beyond mere number generation.
It extends its utility to shuffling lists or selecting random elements from a sequence, proving indispensable for data sampling or when the randomness of a card game shuffle is required.
Such capabilities are particularly useful for selecting random samples from datasets or shuffling cards in a game, demonstrating the module's broad applicability.
The following sections delve into additional utilities provided by the random module.
Shuffling List Elements
The shuffle() method reshuffles the elements of a list, bringing an element of unpredictability to your lists.
Here’s how it works in practice:
import random
# Shuffle a list
list = [1, 2, 3, 4, 5]
random.shuffle(list)
print(list)
This code snippet takes a simple list and randomizes its order, showcasing the shuffle function’s ability to mix elements in a list, such as numbers 1 through 5 in this example.
For example, the list might be reshuffled to:
[2, 4, 1, 5, 3]
The reshuffled list presents a new sequence of the same numbers, offering a fresh order with every execution.
Selecting a Random List Element
The choice() method simplifies the process of selecting a random list element.
import random
# Select a random list element
list = [1, 2, 3, 4, 5]
element = random.choice(list)
print(element)
By employing the random.choice() method, the script randomly picks an element from a predefined list, showcasing the ease with which random selection can be achieved.
A potential outcome might be:
4
Drawing a Random Sample from a List
The sample() method stands out for its ability to extract a specific number of unique elements from a list, facilitating random sampling without replacement.
For instance, selecting three unique elements from a list might result in:
import random
# Draw a sample of 3 elements from the list
list = [1, 2, 3, 4, 5]
sample = random.sample(list, 3)
print(sample)
This functionality is invaluable for scenarios requiring random yet distinct selections, illustrated by an output such as:
[2, 1, 5]
Understanding Probability Distributions
Beyond integer generation and list manipulation, the random module allows for number generation based on specific probability distributions, enriching its utility in scientific modeling and analysis.
This capability is crucial across various scientific domains, where the modeling of natural phenomena demands specific distribution patterns.
Whether it’s generating a number with a uniform distribution or employing a Gaussian distribution for more complex modeling, the random module provides the tools necessary for detailed scientific exploration and simulation.
Here's how you might use these distributions in practice.
This is a uniform distribution.
import random
# Uniform distribution example
uniform = random.uniform(1, 10)
print(uniform)
7.598591990243651
This is a Gaussian distribution.
import random
# Gaussian distribution example
gaussian = random.gauss(0, 1)
print(gaussian)
1.5511074196756371
Each distribution serves its purpose, from simulating equitable chances across a range to mimicking natural variance around a mean, demonstrating the module’s adaptability in representing complex real-world phenomena.
What's the difference between uniform and Gaussian distributions? The uniform distribution assigns the same probability to all values within a defined interval, resulting in a flat, constant appearance; it's as if every number had an equal chance of being drawn in a lottery. The Gaussian or normal distribution, however, concentrates most probabilities around a mean value, with probabilities symmetrically decreasing as you move away from this center, resembling the shape of a bell. This reflects natural situations like the height of individuals in a population, where most people are close to the average height and very few are extremely short or tall.
The Significance of Seed in Random Generation
The "seed" concept introduces predictability into random number generation, enabling the reproduction of specific sequences for consistent testing or simulation outcomes.
Setting a seed guarantees the reproducibility of results, an essential feature for simulations requiring consistent outcomes across multiple runs.
An illustrative example demonstrates the impact of setting a seed:
import random
# Set a seed for reproducibility
random.seed(42)
# Draw a sample of 3 elements from the list
list = [1, 2, 3, 4, 5]
sample = random.sample(list, 3)
print(sample)
Such an approach ensures that the generated sequence remains consistent.
In this script, the random.seed() method sets the seed to the integer number 42.
It then draws a sample of three elements from the list.
For example, it will always be [1, 5, 3].
[1, 5, 3]
By changing the seed value, you can generate different random sequences.
For example, choosing a seed like 31 or 20, instead of 42, will yield another unique and distinct sequence of random numbers compared to other seeds.
The Python random module exemplifies the intricate balance between simplicity and depth, offering tools that extend beyond basic random number generation to facilitate a deeper understanding of mathematical and scientific principles through practical application.
This guide aims to provide a comprehensive overview of the random module's power and versatility, inspiring further exploration and application in your Python projects.