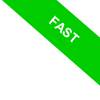
Python's Namespace Packages
Python's namespace packages offer a sophisticated framework for managing "scattered packages," eliminating the need for an `__init__.py` file in every directory.
This ingenious mechanism seamlessly integrates multiple, physically distinct directories as components of a unified Python package.
This capability is invaluable for decomposing a voluminous package into smaller, more digestible segments, or facilitating collaboration among various individuals or teams on different segments of a single Python package.
How Namespace Packages Operate
Through the import command, Python peruses the specified paths in sys.path for modules or packages.
If it stumbles upon a directory missing an `__init__.py` file, it considers this directory as a part of a namespace package, provided there exist other directories under the same name that include parts of the package, even if located in completely different areas.
Let’s walk through a practical example:
Imagine two distinct directories, `/path/to/directory1` and `/another/path/to/directory2`, each containing components of a package dubbed `my_package`.
Here’s what the setup looks like:
/path/to/directory1/my_package/
module1.py
/another/path/to/directory2/my_package/
module2.py
Provided these directories are within the search scope of sys.path, Python will regard them as constituents of a singular namespace package, `my_package`.
In such scenarios, Python forges an implicit namespace package, streamlining the consolidation of modules within this dispersed package architecture.
This integration permits the seamless importation of `module1` and `module2` as though they were elements of a single, cohesive package, despite their residence in separate directories.
Put simply, the import command facilitates the discovery and importation of modules, cataloging directories devoid of an `__init__.py` file and amalgamating them into a namespace package for more efficient access in the future.
Module imports can then be gracefully executed as follows:
from my_package import module1
from my_package import module2
How to Create a Namespace Package
To inaugurate a namespace package, it's crucial to ensure that none of the directories forming the package contain an `__init__.py` file.
This serves as a signal to Python that the directory warrants treatment as part of a namespace package.
Subsequently, each piece of your package can be distributed independently. Once the end user has all the components installed within their `PYTHONPATH`, Python will treat it as a single, integrated package.
To conclude, namespace packages present a powerful and adaptable strategy for the organization and distribution of Python code across several directories.
They are particularly advantageous in large projects or scenarios involving collaboration across different parts of a package. However, it’s wise to be cognizant of the potential for slightly more complex dependency management. Employ this feature judiciously.
This overview should provide a thorough understanding of namespace packages in Python and their practical utility.
If you have further questions or wish to delve deeper, feel free to reach out.