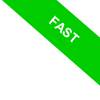
Python's datetime Module
Python's datetime module is a powerhouse for dealing with dates and times, offering a rich array of functions and classes tailored for time management.
Essential for any Python programmer, this module is a go-to resource for creating, manipulating, and formatting date, time, and datetime objects. Its adaptability makes it perfect for navigating the complexities of time-related challenges.
It specifically enables you to craft various types of objects like date, time, datetime, and more, each serving a unique purpose.
- Date Objects
Ideal for representing calendar dates without the time aspect. Think of storing important dates like birthdays or deadlines. - Time Objects
These objects focus solely on time, disregarding the date. They're your tool for recording times such as the start and end of meetings or tasks within a day. - Datetime Objects
A fusion of date and time, these objects are perfect for pinpointing specific moments, complete with both date and time details. - Timedelta Objects
For measuring time spans - the interval between two points in time. They're indispensable for calculating durations. - Timezone Objects
Handling timezone information is a breeze with these objects. They're critical for timezone conversions and managing date-time operations across different time zones, including adjustments for daylight saving time.
Let's dive into some hands-on examples to illustrate their usage.
Start by importing the datetime module:
import datetime
Or, if you prefer, import only the functions you need:
from datetime import date, time, datetime
This approach allows you to use the methods and functions directly, omitting the 'datetime.' prefix. For instance, write date(2024,1,15) instead of datetime.date(2024,1,15).
To instantiate a date object for a specific day, use the date() function.
Simply define it by specifying the year, month, and day:
d = datetime.date(2024, 1, 18)
Create a time object to hold a specific time using the time() function. Just input the hour and minute:
t = datetime.time(12, 30)
For an object encapsulating both date and time, turn to the datetime() function.
dt = datetime.datetime(2024, 1, 18, 12, 30)
These objects are now at your disposal for data processing or scripting purposes.
To add days to a date, for example, use the timedelta() function.
tomorrow = d + datetime.timedelta(days=1)
Calculating the difference between two similar objects is straightforward:
duration = tomorrow - d
print(duration)
1 day, 0:00:00
Extracting and manipulating individual components from these objects is easy with Python's formatting and parsing functions.
To format a date object into a string, for example:
formatted_date = d.strftime("%Y-%m-%d")
print(formatted_date)
'2024-01-18'
Converting a string into a datetime object is just as simple:
from datetime import datetime
date_string = "15 January, 2024"
parsed_date = datetime.strptime(date_string, "%d %B, %Y")
print(parsed_date)
2024-01-15 00:00:00
These examples barely scratch the surface of what's possible. In upcoming tutorials, we'll explore more intricate scenarios, such as managing daylight saving time changes, converting between various time zones, and handling more unconventional date and time formats.