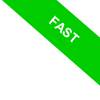
Python Packages
A Python package is essentially a folder packed with multiple code files, or modules, each brimming with its own functions, classes, and variables. It's a modular approach to bundling related functionality together.
To simplify, envision a module as a box within a box scenario. Your package is the big box, and inside it, you place several smaller boxes—your modules. Each of these can contain functions, classes, or variables—pieces of code dedicated to performing specific tasks.
A package is just a special kind of module, one that can contain other modules. But it's important to note that not all modules qualify as packages.
A module earns its package status when it encompasses other modules, which is where the special attribute "__path__" comes into play.
__path__
This attribute is essentially a list of directories where Python looks for any submodules contained within the package.
So, a package can encompass a variety of modules, whether they're located in the same directory or spread across different folders on your system.
The Purpose of a Package: Packages are your best friend when it comes to organizing your code. They allow you to logically structure your code, especially in large projects with numerous functionalities. Cluttering all your code into a single file? That’s a no-go. Packages keep your code neat, readable, and manageable. Moreover, if you have modules of the same name, specifying the package they belong to can save you from confusion.
Let’s walk through a practical example for better understanding.
Imagine you’re spearheading a Python project for a quaint bookshop.
Our project is structured into a package named "bookstore". Within this package are several modules:
- inventory.py: Handles the ins and outs of the shop's book inventory.
- sales.py: Manages sales transactions, discounts, and totals.
- customers.py: Takes care of customer registration and data management.
Each module is tasked with a specific function, be it inventory management, sales processing, or customer relations.
Files like "inventory.py", "sales.py", and "customers.py" are modules because they encapsulate reusable Python code. However, they're not packages since they don’t house other modules.
Our "bookstore" package structure might look something like this:
bookstore/
__init__.py
inventory.py
sales.py
customers.py
Here, "bookstore" is our overarching package, containing several modules (inventory.py, sales.py, customers.py).
The __init__.py file is crucial for Python to recognize the "bookstore" directory as a package. This file can be left empty, but its presence is compulsory.
To leverage the functionalities within these modules, you would import them as follows:
from bookstore import inventory, sales, customers
# Now, access functions and classes from each module becomes straightforward
inventory.add_book('The Old Man and the Sea', 'Ernest Hemingway')
total_sale = sales.calculate_total([{'title': 'The Old Man and the Sea', 'price': 10.00}])
customers.add_customer('John Doe', 'john.doe@email.com')
This example shows "bookstore" as a package because it contains other modules and has the "__path__& quot; attribute, guiding Python on where to find the included modules, specifically in the /bookstore folder.
To direct Python to the correct package, simply use a dot to separate the package name from the module name.
Every line of code mentioned above demonstrates how to invoke a function from a different module, illustrating the seamless interaction between modules within a package.
Example 2
For another angle on the utility of packages, consider this scenario:
If you happen to have a module with the same name in two different directories within Python's search path, specifying which one to import becomes crucial.
Suppose there are two modules both named "mymodule.py", located in "test1" and "test2" folders, respectively.
test1/
__init__.py
mymodule.py
test2/
__init__.py
mymodule.py
By specifying the package upon import, you ensure that Python pulls in the correct module, thus avoiding any potential mix-up.
import test1.mymodule
or
import test2.mymodule
This method clarifies which module you're working with, thanks to the explicit package name.
Python Package Types
In the realm of Python, packages fall into two primary categories:
- Regular Packages
For a package to be considered regular, it must include an `__init__.py` file. This file signals to the Python interpreter that it's dealing with a package, essentially organizing modules under one roof. This structure is ideal when your package’s entire codebase needs to be bundled and distributed as one unit. Picture a suite of modules neatly packed into a single folder—that's your quintessential example of a regular package. - Namespace Packages
On the flip side, namespace packages do away with the need for an `__init__.py` file. This absence allows them to assemble modules from various directories, scattered across different locations. Thanks to their enhanced flexibility, namespace packages simplify the development and distribution process when you’re dealing with pieces of code that belong to the same family but reside in separate folders.
Working with Third-Party Packages in Python
A wide array of packages and applications, crafted by fellow Python enthusiasts, are readily available for your use.
This luxury allows you to integrate pre-built functionalities into your projects, bypassing the need for ground-up development.
A noteworthy distinction lies between packages and applications: the latter come equipped with startup scripts and configuration files.
Before leveraging these resources, their installation within your Python environment is essential.
How to Install Third-Party Packages
The pip package manager is your go-to tool for installing most third-party Python packages with ease.
To install a package, such as "example_package", simply execute the command below in your terminal:
pip install example_package
For example, installing the 'requests' package, which enables HTTP requests, is as straightforward as typing:
pip install requests
Putting Third-Party Packages to Use
Following installation, incorporating a package into your Python script is a matter of importing it.
Whether you're importing an entire package, specific modules, or even particular functions/classes from those modules, the process remains intuitive. Here's how:
To import a whole package:
import example_package
For importing a specific module within a package, append the module name after the package name, separated by a dot:
import example_package.module
And to import just a specific function from a module, the 'from import' syntax is recommended:
from example_package.module import function
A Practical Example
Having installed the 'requests' package, it can be summoned with an import statement and utilized accordingly.
import requests
# Execute a GET request
response = requests.get('https://www.wikipedia.org')
# Display the response's status code
print(f'Status Code: {response.status_code}')
Through this script, the 'requests' package is imported to fetch and store web page content, showcasing the simplicity yet power of Python packages. They expand your programming capabilities without the overhead of starting from zero for each new feature.
This example hopefully clarifies how leveraging Python packages can significantly streamline your coding endeavors.