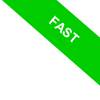
Python Modules
Let's dive right into today's Python tutorial, where we'll be unwrapping the concept of Python modules. We'll explore what they're all about, how to use them, and most importantly, how they can revolutionize your programming experience.
First off, what's a module? In the world of Python, a module is simply a file, brimming with definitions, functions, and instructions. It's a toolbox of functions, if you will. The beauty of modules is that they offer you a chance to leverage existing functions without having to roll up your sleeves and write them all from scratch. Take the "math" module, for example. It's your go-to place for all things mathematical. The "statistics" module? That's where you'll find your statistical functions, and so on. By compartmentalizing your code, modules make it more readable and easier to handle, particularly when you're dealing with large-scale projects.
Modules aren't limited to just function definitions, they can also include classes, variables, and even executable code.
So, why are they useful?
Once you've defined a module, it's yours to call upon in any script.
What's more, you're not just limited to creating your own bespoke modules. You can also make use of those developed by fellow coders in the Python community.
So, how do you get started with modules?
To put a Python module to work, you first need to import it with the import statement.
import module_name
Say you want to use the "math" module, replete with a smorgasbord of handy mathematical functions. Your command would be: import math.
import math
When you import a module, Python transforms it into a bytecode object, making it ready for use.
Once you've got the module on board, you can start calling its functions using dot notation.
For instance, nestled within the "math" module, you'll find a handy function, sqrt(), that's designed to calculate the square root of a number.
Here's how you'd use it: math.sqrt()
- import math
- x = 16
- sqrt_x = math.sqrt(x)
- print(sqrt_x)
This unassuming script calculates the square root of 16.
Voilà! The result is 4.
4.0
Bear in mind, you only need to invoke the import statement once, either at the outset of your code or at the start of your session if you're in the Python console.
Once a module's functions are imported into your program, you can call on them any time without having to repeat the import process.
Consider this example, where the sqrt() function is used twice.
- import math
- x = 16
- sqrt_x = math.sqrt(x)
- print(sqrt_x)
- x = 25
- sqrt_x = math.sqrt(x)
- print(sqrt_x)
You'll notice that the import math statement only makes one appearance at the beginning of the code.
Standard modules and third-party modules
Python has two categories of modules: standard modules and third-party modules.
Standard Modules
Python comes pre-equipped with an extensive standard library of modules. These offer a wealth of additional functionalities for a myriad of tasks.
Take the "math" module for instance, packed with practical mathematical functions. Then there's the "json" module, designed to assist with creating or processing JSON files. And there's more where they came from, with modules for everything from string manipulation to OS access.
To deploy standard modules, you simply import them into your code with the import statement.
import module_name
Beyond standard modules, Python has an ocean of third-party modules available to expand its capabilities even further.
Third-party Modules
To harness the power of third-party modules, you'll need to first install them on your machine using Python's package manager, pip.
Take the "requests" module, a popular third-party tool that makes sending HTTP requests a breeze.
You can install it on your machine with this command:
pip install requests
Once it's installed, you can import it into your Python script just like any other standard module.
- import requests
- response = requests.get('')
- print(response.text)
Crafting Custom Modules
In addition to importing existing modules, Python also gives you the power to create your own custom modules.
Creating a module is as simple as penning a new Python file.
Here's an example:
- def greet(name):
- print(f"Hello, {name}!")
This code houses the definition of the greet() function, which accepts an input parameter and prints out a warm welcome.
Now, save this code in a file with a ".py" extension, say "mymodule.py"
Once saved, you can bring the "mymodule" into a Python script using the import statement.
- import mymodule
- mymodule.greet("Alice")
A module's name is simply the filename without the suffix.
Therefore, it's important to remember not to append the ".py" extension when importing the module into your Python script.
This way, the greet() function from your custom module is ready and waiting in any other script, with no need for rewriting.
In this case, the script prints out the message "Hello, Alice!"
Hello, Alice!
It's a simple example, almost simplistic, but it highlights the potential power of custom modules.
Types of Modules
Modules come in a few different flavors:
- Python Modules
At its core, a Python module is simply a .py file brimming with commands. When you import one, it transforms into bytecode and gets neatly filed away for use. - Compiled Modules
These are files already in bytecode form. They cut straight to the chase by saving directly to your hard drive upon import. Since they're pre-compiled, you can use them right away without any extra steps. - Built-in Modules
The built-in variety is part of Python's standard toolkit. Crafted in C, these modules are pre-compiled and linked right into the Python interpreter, making them ready to roll as soon as you import them. - C/C++ Extensions
For those needing a bit more horsepower, C/C++ extensions offer compiled, dynamically linked muscle. Whether they're Fortran, C, or C++ programs, these are turned into dynamic libraries, coming with .dll or .pyd extensions on Windows and .so on Linux.
In conclusion, Python modules are a potent tool that lets you efficiently organize and reuse your code.
Embracing modules can pave the way for cleaner, more manageable, and reusable code.