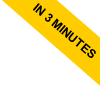
Data Operations in R: Selecting, Filtering and Sorting
Welcome to our R language tutorial where we'll dive into the essentials of data manipulation: selecting, filtering, and sorting data. These powerful tools are pivotal for uncovering hidden insights within datasets.
Purpose: They enable you to delve into the characteristics and relationships buried in data, making analysis both insightful and efficient.
Let's kick things off with a practical example. We'll create a data frame, which will be our playground for these operations.
- df <- data.frame(
- Name = c("Alice", "Bob", "Carla", "David", "Eva"),
- Age = c(25, 35, 45, 20, 30),
- Score = c(88, 92, 95, 70, 85)
- )
Meet "df", our simple yet informative data frame. It consists of three columns and five rows, providing a snapshot of our dataset.
Name | Age | Score |
---|---|---|
Alice | 25 | 88 |
Bob | 35 | 92 |
Carla | 45 | 95 |
David | 20 | 70 |
Eva | 30 | 85 |
With "df" at our disposal, we're set to explore the intricacies of data selection, filtering, and sorting.
Setting Up: Installing the dplyr Library
Before diving in, ensure you have the dplyr library installed in R. It's a suite of functions tailored for efficient data manipulation.
What does dplyr offer? It’s a powerhouse of functions streamlining tasks like filtering, selecting, sorting, and summarizing data. This tutorial will specifically leverage functions like select(), slice(), filter(), and arrange(). It's a cornerstone for data analysis in R.
Installation Guide: If it's not yet in your toolkit, install it with install.packages("dplyr").
install.packages("dplyr")
Once installed, bring it into any R session or script with library("dplyr").
library(dplyr)
Now, you're all set to explore data selection, filtering, and sorting in-depth.
Data Selection: The Art of Extraction
Data selection is all about pinpointing specific columns or rows from a dataset.
Column Selection
The select() command from the dplyr package is your go-to for column extraction.
Let’s say you need just the "Name" column from "df". Here’s how you do it:
df_selected <- select(df, Name)
select() elegantly picks out the "Name" column, storing it in "df_selected".
print(df_selected)
Name
1 Alice
2 Bob
3 Carla
4 David
5 Eva
Need more than one column? No problem. Let's grab both "Name" and "Age" this time.
df_selected <- select(df, Name, Age)
Voilà! "df_selected" now holds two columns.
print(df_selected)
Name Age
1 Alice 25
2 Bob 35
3 Carla 45
4 David 20
5 Eva 30
Row Selection
For row-wise selection, turn to the slice() function of dplyr.
For example, to get the first and third rows of "df", you’d go with:
df_sliced <- slice(df, c(1, 3))
slice() neatly extracts these rows, tucking them into "df_sliced".
print(df_sliced)
Name Age Score
1 Alice 25 88
2 Carla 45 95
Data Filtering: Sifting Through Data
Filtering is akin to a sieve, letting you isolate rows that meet specific criteria.
The filter() function from dplyr is your ally here. Let's filter out rows where Age is over 30.
df_filtered <- filter(df, Age > 30)
This filters and secures the relevant rows in "db_filtered".
print(df_filtered)
Name Age Score
1 Bob 35 92
2 Carla 45 95
Combining criteria? Absolutely. Filter for age ranges, for instance, between 20 and 30.
df_filtered <- filter(df, Age >= 20, Age <= 30)
filter() now hones in on records meeting both age thresholds.
print(df_filtered)
Name Age Score
1 Alice 25 88
2 David 20 70
3 Eva 30 85
Data Sorting: Ordering with Precision
Sorting involves arranging rows in a specified sequence.
The arrange() function from dplyr is perfect for ordering data ascendingly or descendingly.
Ascending Order:
For an ascending sort by Age in "df", use:
df_sorted <- arrange(df, Age)
This sorts "df" by Age, saving the ordered set in "df_sorted".
print(df_sorted)
Name Age Score
1 David 20 70
2 Alice 25 88
3 Eva 30 85
4 Bob 35 92
5 Carla 45 95
Descending Order:
To sort in reverse, simply add the desc() clause to arrange().
df_sorted <- arrange(df, desc(Age))
Now, "df" is ordered from the oldest to youngest.
print(df_sorted)
Name Age Score
1 Carla 45 95
2 Bob 35 92
3 Eva 30 85
4 Alice 25 88
5 David 20 70
Mastering these fundamental operations in R can dramatically enhance your data analysis, offering a myriad of ways to extract and interpret valuable insights.