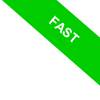
Reading and Writing Files with R
Understanding file input and output in R programming is an essential skill, particularly for those in data analysis. This step-by-step guide will help you navigate these vital functions with ease.
How to Open and Close Files in R
Initiating file interaction in R begins with the file() function. This function requires two essential arguments: the file path and the mode (e.g., 'r' for read, 'w' for write).
fileConn <- file("example.txt", "w")
Completing your read or write tasks demands the proper closure of the file using the close() function, a critical step for resource management.
close(fileConn)
This ensures the system resources used by the file are efficiently released.
Writing Data into a File
With the file opened in write mode ('w'), you can begin data entry using functions such as write.table(), writeLines(), or cat().
- fileConn <- file("example.txt", "w")
- data <- c("Line 1", "Line 2", "Line 3")
- writeLines(data, fileConn)
- close(fileConn)
This R script, composed of four principal commands, efficiently writes data into a text file. Let's delve into each step:
- The script starts by opening "example.txt" in write mode ('w'). If the file doesn't exist, it is automatically created. "fileConn" acts as a handle to this opened file, though you may choose any variable name.
fileConn <- file("example.txt", "w")
- Next, a vector named `data` is created, containing the strings "Line 1", "Line 2", and "Line 3", marking the data you wish to write into the file.
data <- c("Line 1", "Line 2", "Line 3")
- The third step involves writing the `data` vector contents into the file using `fileConn`. Each element of the vector is placed on a separate line, resulting in a three-line text file.
writeLines(data, fileConn)
- Finally, closing the file connection with `close(fileConn)` is an essential step for data integrity and resource allocation.
close(fileConn)
Thus, this script creates "example.txt", populates it with three lines of text, and ensures the file is properly closed afterward.
Opening the file in any text editor will display its contents:
This is a quintessential example of basic file writing operations in R.
Reading Data from a File
Reading data from a file in R requires opening it in 'r' (read) mode and employing functions like read.table(), readLines(), or scan().
- fileConn <- file("example.txt", "r")
- readData <- readLines(fileConn)
- close(fileConn)
Let's break down this straightforward R script for reading data from a text file:
- It begins by establishing a link to "example.txt". `file()` is the R function used for this purpose, and 'r' indicates the read mode.
fileConn <- file("example.txt", "r")
- The second line reads the file content through the established link, storing the data in `readData`. If the file has multiple lines, each is stored as a separate vector element.
readData <- readLines(fileConn)
- The script concludes by closing the file connection with `close(fileConn)`, a necessary step to end the read operation and release the file link.
close(fileConn)
This script exemplifies a professional and efficient approach to reading data from a text file in R, a common practice in various R-based data handling scenarios.
Handling CSV Files
CSV files are ubiquitous in data analysis, and R has tailored functions like read.csv() for seamless CSV file handling.
To read data from a CSV file, say "data.csv", simply input:
dataCsv <- read.csv("data.csv")
Writing data back into a CSV is just as straightforward, utilizing the write.csv() function.
write.csv(dataCsv, "modified_data.csv")
This command effortlessly creates a new file on your computer in your chosen location and directory.
Navigating Paths and Directories with Ease
Knowing your file paths is crucial for error-free file manipulation.
Set your working directory effortlessly with setwd()
setwd("path/to/folder")
To display the current directory path, simply use getwd()
getwd()
This command neatly returns the current path.
[1] "C:/temp"
Error Handling in File Operations
Effective error management is paramount when dealing with file operations.
R's tryCatch() function is a robust tool for preemptively catching errors during file operations.
Let's break down a practical application:
- fileConn <- NULL
- tryCatch({
- # Initiates an attempt to open the file for reading
- fileConn <- file("example.txt", "r")
- readData <- readLines(fileConn)
- close(fileConn)
- }, error = function(err) {
- # Handles any encountered errors during the file's opening or reading process
- print(paste("An error occurred:", err))
- }, finally = {
- # This segment is executed regardless of error occurrence
- if (!is.null(fileConn)) {
- # Verifies if the file connection was established and closes it if so
- try(close(fileConn), silent=TRUE)
- }
- })
Initially, we initialize fileConn as NULL, allowing us to later verify if the file connection was successfully established.
The tryCatch block is utilized to confirm the file's existence:
- try: This block is tasked with opening the file, reading its contents, and closing it afterwards.
- catch: In case of an error during the try block execution (such as the file being non-existent or unreadable), this block takes over. Here, the error is captured and displayed.
- finally: Executed regardless of error occurrence, this block checks if fileConn is open and closes it if necessary. This is vital for preventing resource leakage and ensuring smooth system resource management.
The strategic use of tryCatch enhances the code's resilience to unexpected errors, simplifying exception handling during execution.
In conclusion, deftly managing file operations in R is a blend of attention to detail and practical experience. It's vital to acquaint oneself with the array of functions and the nature of data being processed.
With dedicated practice, you'll soon excel in data reading and writing, an indispensable skill for any data analyst.