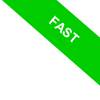
Functional Programming in R
In the world of programming, functional programming stands out as a paradigm where functions take the center stage. R, with its unique language structure, lends itself exceptionally well to this approach.
How does it work? The essence of functional programming lies in replacing traditional loops with function recursion. This means a function repeatedly calls itself. Additionally, it leverages higher-order functions, which are capable of accepting other functions as arguments or returning a function as their outcome.
When delving into functional programming, it's crucial to understand two primary function types:
- Pure Functions
These are the functions that perform mathematical operations and yield a result, steering clear of any side effects like altering global variables or printing to the screen. - Higher-Order Functions
These are advanced functions that can take other functions as parameters, enhancing their utility and flexibility.
Strive to keep your functions as pure as possible and harness the power of higher-order functions for their invocation.
Let's clarify these concepts with some hands-on examples.
Higher-Order Functions
Higher-order functions stand out as they accept other functions as arguments and operate on data. Consider, for instance, the task of doubling each element in a list.
This can be elegantly achieved by combining a pure function with a higher-order function.
- double <- function(x) {
- return(x * 2)
- }
- numbers <- c(1, 2, 3, 4, 5)
- doubled_numbers <- sapply(numbers, double)
- print(doubled_numbers)
Here, sapply is a quintessential higher-order function that takes a list (1, 2, 3, 4, 5) and the double() function as inputs.
It then adeptly applies double() to each element in the list, showcasing the power of higher-order functions.
The result is a neatly doubled list:
2 4 6 8 10
Every element in the list is effectively doubled.
Higher-Order Functions with Anonymous Functions
Adding another layer of sophistication, higher-order functions can also employ anonymous functions as arguments.
Here's a practical application:
- numbers <- c(1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
- even_numbers <- Filter(function(x) x %% 2 == 0, numbers)
- print(even_numbers)
This script uses an anonymous function to sift through and identify even numbers.
The Filter function, a higher-order function, then takes this anonymous function and the number list, effectively isolating and displaying the even numbers.
2 4 6 8 10
This serves as a textbook example of how higher-order functions can be applied.
Recursion
Recursion is a technique where a function calls itself as part of its process. This approach is invaluable for breaking down complex problems into more manageable sub-problems.
Consider the task of calculating a number's factorial, a classic scenario for employing recursion.
- factorial <- function(n) {
- if (n == 0) {
- return(1)
- } else {
- return(n * factorial(n - 1))
- }
- }
- print(factorial(5))
In this example, the factorial function continues to invoke itself until it reaches the base condition (n == 0).
Upon reaching this point, it sequentially multiplies the results from previous iterations.
The end result is an elegant computation of the factorial of five, equating to 120.
120
Functional programming in R might seem abstract at first glance, but it's a powerful paradigm for crafting clear and concise code. Exploring the realms of pure functions, recursion, and higher-order functions can significantly boost your proficiency in writing efficient R programs.