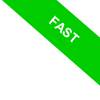
Debugging and Error Handling in the R Language
This lesson delves into the nuances of debugging and error handling within the R language.
What exactly is debugging? Imagine a programming language as your data experimentation playground. Just like in a physical lab, you need various tools for measurement and analysis, and sometimes, things don't go as planned. In the realm of programming, these tools are your debugging techniques.
When you stumble upon an error, take a moment to thoroughly read the message. R is trying to communicate the problem to you. It's akin to an experiment deviating from its expected course: don't just brush it off, but rather, pay attention to what it's telling you.
For example, encountering an error like the one below indicates that you're attempting to use a non-existent object `y` in your workspace.
Error in funzione(x) : object 'y' not found
Luckily, R is equipped with an array of debugging tools to help you navigate and resolve issues in your code.
Let's explore some practical debugging strategies in R.
Monitoring Variable States During Execution
A straightforward yet powerful approach to troubleshooting is to use the print() statement for monitoring variable values at various stages of your script's execution.
Consider it akin to placing checkpoints in your code, helping you pinpoint where things might be going awry.
If a segment of your code is malfunctioning, inserting a print(variable_name) can reveal the value of that variable at that specific moment.
Consider this code snippet with an embedded error.
- calculate_average <- function(numbers) {
- sum <- sum(numbers)
- length <- length(numbers)
- average <- sum / length
- return(average)
- }
- # Note: "4" is actually a string, not a number.
- numbers <- c(1, 2, 3, "4", 5)
- calculated_average <- calculate_average(numbers)
- print("Computed Average:")
- print(calculated_average)
Executing this script results in an error due to one of the elements in the 'numbers' array being a string, disrupting the average calculation process.
Error in sum(numbers) : invalid 'type' (character) argument
If the cause of the problem isn't immediately clear from the error message, strategically placed print() statements can aid in diagnosing the issue during script execution.
- calculate_average <- function(numbers) {
- print("Input Values:")
- print(numbers)
- sum <- sum(numbers)
- print("Sum Calculated:")
- print(sum)
- length <- length(numbers)
- average <- sum / length
- return(average)
- }
- numbers <- c(1, 2, 3, "4", 5)
- calculated_average <- calculate_average(numbers)
- print("Computed Average:")
- print(calculated_average)
This approach reveals the variable states at critical junctures, aiding in identifying the root cause within the calcola_media function.
[1] "Input Values:"
[1] 1 2 3 "4" 5
Error in sum(numeri) : invalid 'type' (character) argument
This observation confirms that the "numeri" array includes a string, causing the calculation discrepancy.
Pausing Script Execution
An invaluable technique for deeper analysis involves the use of the browser() function.
This R language tool effectively pauses your script midway, offering a chance to explore your working environment in depth.
Essentially, it suspends execution without a complete halt, allowing step-by-step progression and variable value examination. It's comparable to momentarily freezing time in your lab to scrutinize every detail at leisure.
For instance, inserting a browser() at a suspected problem area in your script pauses execution right there. You're then free to run R commands to inspect variables.
- calculate_sum <- function(a, b) {
- # Place browser() here
- browser()
- result <- a + b
- return(result)
- }
- a <- 5
- b <- "3"
- sum <- calculae_sum(a, b)
- print(sum)
On executing this script, the code pauses at the browser() insertion point, and a special "Browse" prompt appears in the terminal.
Browse[1]>
You now enter an interactive debugging session, where you can:
- Press n and enter to advance to the next code line.
- Enter a variable name to view its current value.
- Execute additional R commands to test your hypotheses regarding the issue.
For instance, typing a and b would reveal their values, and you'd discern that b is a string "3", which explains the malfunction in the sum operation.
Browse[1]> a
[1] 5
Browse[1]> b
[1] "3"
Once your investigation concludes, typing c continues the script's normal execution.
The browser() function is invaluable for a closer look at your code, much like an experimental physicist examines their instruments to better understand an observed phenomenon.
Error Handling
There are scenarios where you'd want your script to proceed despite encountering errors.
In such cases, structures like try() or tryCatch() are your go-to solutions.
These constructs execute a code block and gracefully handle any emerging errors, ensuring uninterrupted script execution.
Essentially, they execute the designated code or function and, if an error arises, manage it without halting the entire script.
Imagine a function prone to errors, such as one dividing two numbers, where occasionally the divisor might be a string, leading to a calculation error.
- divide <- function(a, b) {
- return(a / b)
- }
- # Run the function with try
- result <- try(divide(10, "2"))
- if (inherits(result, "try-error")) {
- print("An error was encountered during division")
- } else {
- print(result)
- }
- print("Program completed")
In this scenario, try(divide(10, "2")) attempts the division. If an error occurs, such as division by a string, the try statement captures it instead of halting the entire script.
By using the inherits function, you can determine if "result" represents an error and address it appropriately.
Error in a/b : non-numeric argument to binary operator
[1] "An error was encountered during division"
[1] "Program completed"
As demonstrated, the script continues its execution after handling the error, concluding with "Program completed".
Using tryCatch()
The tryCatch() function offers a more sophisticated approach, enabling tailored responses to different error types.
- result <- tryCatch({
- divide(10, 0)
- }, warning = function(w) {
- print("A warning has been issued")
- }, error = function(e) {
- print("An error has been detected")
- NA # Return NA in case of error
- }, finally = {
- print("Division operation attempted")
- })
- print(result)
Here, tryCatch() tries to execute the division. If an error happens, the designated function under the `error` argument is triggered, issuing a message and returning `NA`.
It's important to note that the finally argument is executed regardless of error occurrence, ensuring a thorough completion check.
[1] "An error has been detected"
[1] "Division operation attempted"
[1] NA
To wrap up, mastering debugging in R is akin to honing scientific exploration skills: it requires patience, a keen sense of curiosity, and a structured approach.
Every error is not just a setback, but a valuable learning opportunity. Embrace these tools and techniques, and you'll gradually become more proficient in detecting and resolving code-related issues.