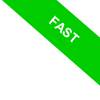
Defining Functions in R
Developing proficiency in writing functions in R is a cornerstone skill for streamlining and enhancing your data analysis processes.
Understanding Functions: Functions are discrete blocks of code, each designed to carry out a specific task, ready for reuse.
Begin by clarifying the function's purpose. Clearly outline the necessary inputs and the expected output.
Crafting a Function
In R, a function is crafted using the syntax:
- myFunction <- function(argument1, argument2, ...) {
- # Body of the function
- }
Arguments are the values passed to the function, and you can incorporate any number of them.
You can also set default values for these arguments, which will be used unless specified otherwise.
- myFunction <- function(a = 1, b = 2) {
- # Body of the function
- }
The function body is where the actual task gets executed. This can involve loops, conditional statements, and invoking other functions, among other elements.
The return() statement within the function body signals the result, or return value, that the function outputs.
- myFunction <- function(a, b) {
- result <- a + b
- return(result)
- }
If the return() statement isn't explicitly used, R automatically returns the last value computed in the function.
Function Invocation
Having written your function, it can be invoked from any part of your program using the following structure:
- myFunction(a, b)
Ensure that the number of parameters corresponds with those defined in your function. Here, we have two parameters: "a" and "b".
Testing it with a variety of inputs is vital to verify its functionality.
Good practice dictates commenting your code and providing a succinct description at the start of each function. This should explain the function's purpose, the arguments it requires, and the type of output it produces. Note: In R, comments are initiated with the # character.
Example in Action
Consider a function designed to calculate the average of two numbers:
- calculateAverage <- function(num1, num2) {
- # Calculation: Sum divided by 2 for the average
- average <- (num1 + num2) / 2
- # Outputting the result
- return(average)
- }
This function receives two numbers as input and outputs their average.
Calling this function from different parts of your program with varying inputs helps avoid redundancy in your code.
Here's how the program looks with three separate function calls:
- calculateAverage <- function(num1, num2) {
- # Calculation: Sum divided by 2 for the average
- average <- (num1 + num2) / 2
- # Outputting the result
- return(average)
- }
- # First invocation
- result1 <- calculateAverage(4, 8)
- print(result1)
- # Second invocation
- result1 <- calculateAverage(5.5, 7.3)
- print(result1)
- # Third invocation
- result1 <- calculateAverage(-10, 20)
- print(result1)
The first function call takes two integers, 4 and 8, and computes their average.
The function duly returns an average value of 6.
6
In the second call, it handles two decimal numbers, 5.5 and 7.3, and calculates the average, which is about 6.4.
6.4
The final call uses the numbers -10 and 20 as inputs, with the function outputting the average as 5.
5
This example illustrates how R functions can bring organization, efficiency, and readability to your coding endeavors.
Handling Array Arguments in R Functions
In R, you have the flexibility to pass an array of values as a function argument. Let's explore how this works with a hands-on example.
Here's a real-world example for clarity.
- # Defining the function
- calculateAverage <- function(numbers) {
- total <- sum(numbers)
- count <- length(numbers)
- average <- total / count
- return(average)
- }
- # Function test with a numeric vector
- testNumbers <- c(1, 2, 3, 4, 5)
- calculatedAverage <- calculateAverage(testNumbers)
- # Result output
- print(calculatedAverage)
In this demonstration, the "calculateAverage" function accepts a numeric vector, tallies their sum, and divides this total by the vector's length to yield the average.
When invoked with the array (1,2,3,4,5), the function should rightly return 3, the average of these figures.
3
This method is an effective way to compute averages for any number of elements.
Before concluding, a few practical tips. Opt for clear, descriptive argument names to enhance your code's readability. Always verify the validity of arguments passed to the function to prevent errors like division by zero. Also, use comments to explain any part of your code that may not be immediately intuitive to others.
Remember, proficiency comes with practice. Continue honing your skills by writing and refining your functions.