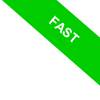
Exploring apply, lapply, and sapply in R
The functions apply, lapply, and sapply are cornerstones of the R programming language, offering vital tools for data manipulation. They shine when it comes to applying a function across each element of various data structures like arrays, matrices, vectors, and lists with efficiency and elegance.
Their Role: These functions are a boon for programmers, allowing the circumvention of explicit loops (such as 'for' or 'while'), leading to more streamlined and tidy code. Essentially, they offer a more sophisticated and readable approach to writing R code.
Let's examine them more closely.
Apply
The apply function is adept at applying a specific function to either the rows or columns of an array or matrix, referred to as X.
apply(X, MARGIN, FUN, ...)
Key parameters of this function include:
- X, representing an array or matrix.
- MARGIN, which determines the focus on rows (1) or columns (2).
- FUN, the function you intend to apply.
Apply function executes the chosen function on the matrix elements and delivers the results.
Utility: This function facilitates operations on every row or column of a matrix, avoiding the need for explicit looping. It embodies its name, "apply", representing the act of applying a function seamlessly, making the code more compact and reader-friendly.
Consider a real-world example:
Create a 3x3 matrix.
matrix <- matrix(1:9, nrow = 3)
When printed, it reveals a structured matrix with three rows and columns:
print(matrix)
[,1] [,2] [,3]
[1,] 1 4 7
[2,] 2 5 8
[3,] 3 6 9
For instance, to calculate the mean of each row:
Use apply with margin 1 (rows) and the function mean().
apply(matrix, 1, mean)
The mean() function computes the average for each row.
[1] 4 5 6
Another scenario:
To sum the elements of each column:
Employ apply with the sum() function and margin 2 (columns).
apply(matrix, 2, sum)
The sum function aggregates the values across each column.
[1] 6 15 24
Lapply
When it comes to lists, the lapply function is your go-to tool.
lapply(List, FUN, ...)
Its parameters are straightforward:
- List, the target list for the function application.
- FUN, the function you wish to execute.
Lapply takes a list and applies a function to each of its components, always returning a list in the end. This characteristic gives rise to its name "lapply" or "list apply."
This functionality ensures the output is always a list, contributing to its efficacy and consistency.
Here's a practical illustration:
Create a list with five elements.
my_list <- list(5, 10, 15, 20, 25)
To compute the square of each element, use lapply with a simple anonymous function.
lapply(my_list, function(x) x^2)
This operation squares each list element, producing a new list with the results.
[[1]]
[1] 25
[[2]]
[1] 100
[[3]]
[1] 225
[[4]]
[1] 400
[[5]]
[1] 625
It's important to note that the original list remains unaltered; lapply generates a new list as its output.
Sapply
The sapply function is a nuanced version of lapply, designed to refine the output for efficiency and clarity.
sapply(X, FUN, ..., simplify = TRUE)
This versatile function incorporates three key parameters:
- X represents a list, vector, or any object you're applying the function to.
- FUN signifies the function you're applying to each element within X. This could be a standard R function like sum or mean, or a custom function you've created.
- ... (ellipsis) stands for additional parameters that can be fed into the function.
- simplify is a boolean toggle that decides if sapply should streamline the final outcome (TRUE) or leave it as is (FALSE). Its default setting is TRUE.
sapply efficiently processes each element, applies the specified function, and delivers a refined result.
What's its Function? The 's' in sapply stands for "simplify," aptly named as it seeks to condense the results produced by "apply." Its main use is to apply a function to every element in a list or vector, then, if feasible, condense those results into a more manageable vector or matrix form. sapply shines when you're aiming for a more streamlined output.
Consider, for example, a list containing five elements.
my_list <- list(5, 10, 15, 20, 25)
Now, let's square each element in the list using sapply coupled with an anonymous function.
sapply(my_list, function(x) x^2)
The outcome is a neatly arranged array:
[1] 25 100 225 400 625
sapply is especially valuable when the goal is to transform the results into a more compact and user-friendly data format than the original.
Choosing between apply, lapply, and sapply
When to best use each of these? apply is optimal for matrix operations.
lapply is your go-to when dealing with lists, particularly if you wish to retain the list structure in the output.
sapply is the preferred choice for a condensed, more approachable output.
Bear in mind that using sapply with outputs of varying lengths might yield unexpected results. In such instances, lapply is a more reliable alternative. For more intricate tasks, there's vapply, which provides greater control over output types. It allows you to predefine the output structure, a topic we'll delve into in an upcoming tutorial.
Experimenting with these functions, applying them to your data sets, and understanding their nuances will streamline your R programming, making your workflow both simpler and more effective.