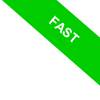
Scoping, Global and Local Variables in R
Scoping in the R programming language is a fundamental concept that significantly influences the interaction between variables and functions across different environments.
What Exactly is Scoping? Scoping, a pivotal programming concept, governs the accessibility of variables and functions within your code. This is particularly vital in R, as it directly impacts data and function management during code execution.
In R programming, variable lookup follows an environment chain, starting from local and extending to global. If a variable isn't found, the program throws an error.
Understanding the distinction between local and global variables is crucial.
Local Variables
Local variables are those that are confined within the functions where they are declared.
These variables remain inaccessible to both the calling program and other functions.
Consider a function designed to calculate a number's square:
calculateSquare <- function(x) {
return(x^2)
}
y <- calculateSquare(3)
print(y)
Here, `x` is a local variable defined within the `calculateSquare` function's environment, confined to that specific context.
Attempting to access `x` outside its function results in an "object 'x' not found" error.
Global Variables
Conversely, global variables are defined in the main program and are accessible throughout, including within any called functions.
For instance, consider a program calculating the square of a global variable, z:
calculateSquare <- function() {
return(z^2)
}
z = 5
y <- calculateSquare()
print(y)
In this scenario, z is a global variable set at 5, and the function returns z squared, yielding a final result of 25.
25
This illustrates how global variables like z can be accessed and manipulated by functions within the program.
However, if a function alters the value of a global variable, it temporarily behaves as a local variable within that function's scope.
Consider this example:
calculateSquare <- function() {
z = z + 1
return(z^2)
}
z = 5
y <- calculateSquare()
print(y)
print(z)
Here, the function modifies z (z = z + 1), making z = 6 within its scope. Consequently, there are two versions of z: the local z = 6 and the original global z = 5.
The function computes the square of 6 (36), but once control returns to the main program, the global z remains unchanged at 5.
36
5
This demonstrates that the function's local changes to a global variable do not affect its global value.
Closures in R
R's lexical scoping enables a unique feature: the creation of "closures".
A closure is a function paired with the environment in which it was defined, allowing it to retain the state of that environment even after the outer function has completed.
Here's an illustrative example:
- createAddition <- function(value) {
- return(function(x) x + value)
- }
- addTwo <- createAddition(2)
- addFive <- createAddition(5)
- print(addTwo(3))
- print(addFive(3))
`addTwo` and `addFive` are closures that "remember" the specific value present at their creation.
`addTwo`, for instance, is a closure born from `createAddition`. It adds 2 to any number passed to it. When you call `addTwo(3)`, it effectively executes the inner function defined in `createAddition` with x = 3 and value = 2, resulting in a sum of 5.
5
Similarly, `addFive` is another closure from `createAddition`, designed to add 5 to any given number. Hence, calling `addFive(3)` yields a total of 8.
8
This is a prime example of how in programming, especially in R, practice and experimentation are key to mastering concepts like scoping. By trying out real-world examples, you can gain a deeper understanding of how scoping influences the functionality of your code.
I hope this guide has provided you with a clearer understanding of scoping in R. If you have any further questions or are curious about other programming topics, feel free to ask. I'm here to help.