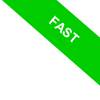
Deleting a row or column in a matrix on Matlab
In this lesson, I'll explain how to remove a row or a column from a matrix in the Matlab environment.
Here's a practical example.
Create a 3x3 matrix with three rows and three columns
>> M=[1 2 3; 4 5 6; 7 8 9]
M =
1 2 3
4 5 6
7 8 9
To remove the first row, type M(1,:)=[ ]
>> M(1,:)=[ ]
M =
4 5 6
7 8 9
What does this command mean?
- The number 1 in the first parameter M(1,:) selects the first row of the matrix
- The colon symbol (:) in the second parameter M(1,:) selects all the columns
Therefore, the command M(1,:)=[ ] selects all the columns of the first row and assigns a null value [ ].
$$ \begin{pmatrix} \not{1} & \not{2} & \not{3} \\ 4 & 5 & 6 \\ 7 & 8 & 9 \end{pmatrix} $$
The first row of the matrix is deleted.
$$ \begin{pmatrix} 4 & 5 & 6 \\ 7 & 8 & 9 \end{pmatrix} $$
The result is a 2x3 matrix with two rows and three columns.
Note. To delete other rows, just indicate a different row number. For example, to remove the second row of the matrix, type M(2,:)=[ ], and so on. The first row of the matrix has an index number equal to 1, the second row equal to 2, etc.
Now create the 3x3 matrix again.
>> M=[1 2 3; 4 5 6; 7 8 9]
M =
1 2 3
4 5 6
7 8 9
To remove the third column of the matrix, type M(:,3)=[ ]
>> M(:,3)=[ ]
M =
1 2
4 5
7 8
This command selects all the rows (:) of the third column and assigns a null value to them.
$$ \begin{pmatrix} 1 & 2 & \not{3} \\ 4 & 5 & \not{6} \\ 7 & 8 & \not{9} \end{pmatrix} $$
The third column is deleted.
$$ \begin{pmatrix} 1 & 2 \\ 4 & 5 \\ 7 & 8 \end{pmatrix} $$
The final result is a 3x2 rectangular matrix with three rows and two columns.
How to delete multiple rows or columns at the same time?
If you want to delete multiple rows or columns in the matrix, you must indicate the list of columns or rows you want to remove in square brackets.
For example, create a 4x4 matrix:
>> M=[1 2 3 4; 5 6 7 8; 9 8 7 6; 5 4 3 2]
M =
1 2 3 4
5 6 7 8
9 8 7 6
5 4 3 2
To delete the first and last rows, type the command M([1 4] ,:)=[ ]
The list of rows to be removed should be written in square brackets [ 1 4 ] in the first parameter of the command.
Separate the row numbers from
>> M([1 4] ,:)=[ ]
M =
5 6 7 8
9 8 7 6
The command M([1 4],:)=[ ] removes the first and third row from the matrix.
$$ \begin{pmatrix} \not{1} & \not{2} & \not{3} & \not{4} \\ 5 & 6 & 7 & 8 \\ 9 & 8 & 7 & 6 \\ \not{5} & \not{4} & \not{3} & \not{2} \end{pmatrix} $$
The result is a 2x4 rectangular matrix with two rows and four columns.
$$ \begin{pmatrix} 5 & 6 & 7 & 8 \\ 9 & 8 & 7 & 6 \end{pmatrix} $$
Now to delete the second, third, and fourth column, type the command M(:,[2 3 4])=[ ]
In this case, the list of columns to be removed [ 2 3 4 ] consists of three columns.
>> M(:,[2 3 4])=[ ]
M =
5
9
The command M(:,[2 3 4])=[ ] removes the second, third, and fourth column from the matrix:
$$ \begin{pmatrix} 5 & \not{6} & \not{7} & \not{6} \\ 9 & \not{8} & \not{7} & \not{6} \end{pmatrix} $$
The result is a 2x1 matrix, or a column vector.
$$ \begin{pmatrix} 5 \\ 6 \end{pmatrix} $$
Before finishing, I'll leave you with one last practical tip.
When the rows or columns are continuous, you can also indicate the interval between the first and last by inserting a colon (:) as a separator, instead of listing them all in a list.
For example, to delete the second, third, and fourth column, you can also write M(:,[2:4])=[ ]
In this case, you are indicating the interval between the columns [2:4] instead of the list [2 3 4].
>> M(:,[2:4])=[ ]
M =
5
9
The final result is still the same.
This way, you can delete any row or column of a matrix when using Matlab.