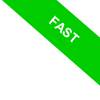
Extracting all diagonals of a matrix in Matlab
In this tutorial, we will explore how to extract all diagonals of a matrix using Matlab.
What are the diagonals of a matrix? Diagonals are the elements of a matrix taken diagonally. For example, the main diagonal of this matrix is the set of elements 1, 5, 9 highlighted in red. $$ M = \begin{pmatrix} \color{red}1 & 2 & 3 \\ 4 & \color{red}5 & 6 \\ 7 & 8 & \color{red}9 \end{pmatrix} $$ The secondary diagonal is instead the set of elements 3, 5, 7 taken in the opposite direction $$ M = \begin{pmatrix} 1 & 2 & \color{red}3 \\ 4 & \color{red}5 & 6 \\ \color{red}7 & 8 & 9 \end{pmatrix} $$
Here's a practical example.
Create a 3x3 square matrix with three rows and three columns.
>> M=[1 2 3 ; 4 5 6 ; 7 8 9]
M =
1 2 3
4 5 6
7 8 9
Type spdiags() to extract all diagonals from the matrix.
>> spdiags(M)
ans =
7 4 1 0 0
0 8 5 2 0
0 0 9 6 3
This command extracts all diagonals of the matrix in addition to the main diagonal and arranges them in a column.
For example, the middle column of the output table (highlighted in red) is the main diagonal of the matrix.
The columns adjacent to the middle column are the diagonals above (green) and below (blue) the main diagonal.
How to extract only one diagonal?
If you want to extract only one diagonal from the matrix, also specify the second parameter of the spdiags() function.
For example, type spdiags(M,0) to extract only the main diagonal of the matrix.
>> spdiags(M,0)
ans =
1
5
9
In the output table of the spdiags() function, the main diagonal of the matrix has index zero (0).
The diagonals above and below the main diagonal, instead, have indices +1 and -1 respectively.
For example, type spdiags(M,1) to extract the diagonal above the main diagonal
>> spdiags(M,1)
ans =
0
2
6
To extract the diagonal below the main diagonal, instead, type spdiags(M,-1)
>> spdiags(M,-1)
ans =
4
8
0
If you want to individually extract the other diagonals, use indices -2, +2 and so on.
How to extract the secondary diagonals?
To extract the secondary diagonals of the matrix M, which go down from right to left, combine the spdiags() function with the fliplr() function that flips the matrix M from left to right.
For example, type spdiags(fliplr(M)) to extract all the secondary diagonals of the matrix.
>> spdiags(fliplr(M))
ans =
9 6 3 0 0
0 8 5 2 0
0 0 7 4 1
The function returns the secondary diagonals of the matrix arranged in a column.
Each column of the matrix is a diagonal of the matrix from left to right.
The central column (red) is the secondary diagonal of the matrix.
The adjacent columns (blue and green) are respectively the diagonals below and above the secondary diagonal.
Note. As in the case of the main diagonal, the central diagonal has index zero (0), the adjacent diagonals have indices -1 and +1, -2 and +2, and so on.
Therefore, to extract only the secondary diagonal, you need to type spdiags(fliplr(M),0). If you want to extract the diagonal above the secondary diagonal, type spdiags(fliplr(M),1), and so on.