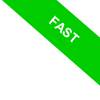
Matrix Operations with Matlab
In this lesson, I will explain how to perform matrix operations using Matlab.
First, create a square matrix M1 with two rows and two columns.
>> M1=[1 4;2 3]
M 1 =
1 4
2 3
Next, create another square matrix M2 with two rows and two columns.
>> M2=[3 1;7 5]
M2 =
3 1
7 5
Now, with these two matrices M1 and M2, let's go through some practical examples of matrix calculations.
- Matrix Addition
- Matrix Subtraction
- Matrix Multiplication
- Element-wise Matrix Multiplication
- Multiplying a matrix by a scalar
- Matrix division
- Element-wise matrix division
- Dividing a matrix by a scalar
- Element-wise matrix exponentiation
- Matrix determinant
- Matrix rank
- Matrix trace
- Transpose of a Matrix
- Matrix inverse
- The Characteristic Polynomial
Matrix Addition
To perform matrix addition, use the plus operator (+).
Type M1+M2
>> M1+M2
ans =
4 5
9 8
$$ M1 + M2 = \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} + \begin{pmatrix} 3 & 1 \\ 7 & 5 \end{pmatrix} = \begin{pmatrix} 1+3 & 4+1 \\ 2+7 & 3+5 \end{pmatrix} = \begin{pmatrix} 4 & 5 \\ 9 & 8 \end{pmatrix} $$
Matrix Subtraction
To perform matrix subtraction, use the minus operator (-).
Type M1-M2
>> M1-M2
ans =
-2 3
-5 -2
$$ M1 - M2 = \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} - \begin{pmatrix} 3 & 1 \\ 7 & 5 \end{pmatrix} = \begin{pmatrix} 1-3 & 4-1 \\ 2-7 & 3-5 \end{pmatrix} = \begin{pmatrix} -2 & 3 \\ -5 & -2 \end{pmatrix} $$
Matrix Multiplication
To perform matrix multiplication, use the multiplication operator (*).
Type M1*M2
>> M1*M2
ans =
31 21
27 17
$$ M1 \cdot M2 = \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} \cdot \begin{pmatrix} 3 & 1 \\ 7 & 5 \end{pmatrix} = \begin{pmatrix} 1 \cdot 3 + 4 \cdot 7 & 1 \cdot 1 + 4 \cdot 5 \\ 2 \cdot 3 + 3 \cdot 7 & 2 \cdot 1 + 3 \cdot 5 \end{pmatrix} = \begin{pmatrix} 31 & 21 \\ 27 & 17 \end{pmatrix} $$
Note that matrix multiplication is called row-by-column multiplication.
You can only perform matrix multiplication between two matrices if the number of columns in the first matrix (M1) is equal to the number of rows in the second matrix (M2).
Element-wise Matrix Multiplication
Element-wise matrix multiplication calculates the product of elements that are in the same position.
It is a different type of matrix multiplication compared to row-by-column multiplication.
To perform element-wise multiplication, use the dot multiplication operator (.*).
>> M1 .* M2
ans =
3 4
14 15
In element-wise multiplication, both matrices must have the same number of rows and columns.
$$ M1 \ .* \ M2 = \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} \ .* \ \begin{pmatrix} 3 & 1 \\ 7 & 5 \end{pmatrix} = \begin{pmatrix} 1 \cdot 3 & 4 \cdot 1 \\ 2 \cdot 7 & 3 \cdot 5 \end{pmatrix} = \begin{pmatrix} 3 & 4 \\ 14 & 15 \end{pmatrix} $$
Multiplying a matrix by a scalar
To calculate the product of a matrix and a scalar, use the multiplication operator (*).
For example, enter 2*M1
>> 2*M1
ans =
2 8
4 6
The elements of the matrix are multiplied by the scalar number 2.
$$ 2 \cdot M1 = 2 \cdot \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} = \begin{pmatrix} 2 \cdot 1 & 2 \cdot 4 \\2 \cdot 2 & 2 \cdot 3 \end{pmatrix} = \begin{pmatrix} 2 & 8 \\4 & 6 \end{pmatrix} $$
Matrix division
Matrix division can be achieved by multiplying the first matrix by the inverse matrix of the second, M1·M2-1.
To calculate the division of two matrices in Matlab, enter M1*inv(M2)
>> M1*inv(M2)
ans =
-2.87500 1.37500
-1.37500 0.87500
Alternatively, you can also enter M1/M2
In this case, Matlab automatically performs the inversion of the second matrix.
>> M1/M2
ans =
-2.87500 1.37500
-1.37500 0.87500
The final result is always the same.
Element-wise matrix division
Element-wise division calculates the quotient between elements that are in the same position.
It is another type of matrix division.
To perform element-wise division, use the operator ./
>> M1 ./ M2
ans =
0.33333 4.0000
0.28571 0.6000
In the case of element-wise division, the two matrices must have the same number of rows and columns.
$$ M1 \ ./ \ M2 = \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} \ ./ \ \begin{pmatrix} 3 & 1 \\ 7 & 5 \end{pmatrix} = \begin{pmatrix} \frac{1}{3} & \frac{4}{1} \\ \frac{2}{7} & \frac{3}{5} \end{pmatrix} = \begin{pmatrix} 0.33333 & 0.28571 \\ 4 & 0.6 \end{pmatrix} $$
Dividing a matrix by a scalar
Dividing a matrix by a scalar is done using the division operator (/).
For example, to divide matrix M1 by two, enter M1/2
>> M1/2
ans =
0.50000 2.00000
1.00000 1.50000
All elements of matrix M1 are divided by the scalar number 2.
$$ \frac{M1}{2} = \frac{1}{2} \cdot \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} = \begin{pmatrix} \frac{1}{2} \cdot 1 & \frac{1}{2} \cdot 4 \\ \frac{1}{2} \cdot 2 & \frac{1}{2} \cdot 3 \end{pmatrix} = \begin{pmatrix} 0.5 & 2 \\ 1 & 1.5 \end{pmatrix} $$
Element-wise matrix exponentiation
To raise every element of a matrix to the same
For example, to raise the elements of matrix M1 to the power of 2, type M1.^2
>> M1.^2
ans =
1 16
4 9
$$ M1 \ \text{.^} \ 2 = \begin{pmatrix} 1^2 & 4^2 \\ 2^2 & 3^2 \end{pmatrix} = \begin{pmatrix} 1 & 16 \\ 4 & 9 \end{pmatrix} $$
Matrix determinant
Matlab has a specific function to calculate the determinant of a square matrix. It is the det() function.
For example, to calculate the determinant of matrix M1, type det(M1)
>> det(M1)
ans = -5
$$ \text{det} (M1) = det \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} = 1 \cdot 3 - 4 \cdot 2 = -5 $$
Matrix rank
To find the rank of a matrix, use the rank() function.
For example, to calculate the rank of matrix M1, type rank(M1)
>> rank(M1)
ans = 2
The rank is equal to 2 because the determinant of the 2x2 matrix is not zero. $$ \text{det} (M1) = det \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} = 1 \cdot 3 - 4 \cdot 2 = -5 $$
Matrix trace
To calculate the trace of a matrix, use the trace() function.
For example, to calculate the trace of matrix M1, type trace(M1)
>> trace(M1)
ans = 4
The trace of a matrix is equal to the sum of the elements on the main diagonal. $$ \text{trace} (M1) = \text{trace} \begin{pmatrix} \color{red}1 & 4 \\ 2 & \color{red}3 \end{pmatrix} = 1 + 3 = 4 $$
Transpose of a Matrix
To transpose the rows and columns of a matrix, use the transpose() function.
For example, to transpose matrix M1, type transpose(M1)
>> transpose(M1)
ans =
1 2
4 3
Alternatively, you can use the matrix transpose operator by adding an apostrophe after the name of the matrix.
>> M1'
ans =
1 2
4 3
In a transpose of matrix, the rows of the matrix become columns and vice versa. $$ \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix}^T = \begin{pmatrix} 1 & 2 \\ 4 & 3 \end{pmatrix} $$
Matrix inverse
To calculate the inverse of a matrix, use the inv() function.
For example, to calculate the inverse of matrix M1, type inv(M1)
>> inv(M1)
ans =
-0.60000 0.80000
0.40000 -0.20000
The inverse of matrix M1 is a matrix that, when multiplied by M1, results in an identity matrix. An identity matrix is a matrix with elements equal to 1 on the main diagonal and 0 elsewhere. $$ M1 \cdot \text{inv} (M1) = \begin{pmatrix} 1 & 4 \\ 2 & 3 \end{pmatrix} \cdot \begin{pmatrix} -0.6 & 0.8 \\ 0.4 & -0.2 \end{pmatrix} = \begin{pmatrix} 1 & 0 \\ 0 & 1 \end{pmatrix} $$
The Characteristic Polynomial
To calculate the characteristic polynomial of a square matrix, you can use the poly() function.
>> poly(M1)
ans =
1 -4 -5