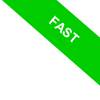
Replacing a Row or Column in a Matlab Matrix
In this lesson, I'll explain how to modify a row or column in a matrix in Matlab, without changing the other rows and columns.
How to replace a row in the matrix
First, create a 3x3 square matrix with three rows and three columns:
>> matrix = [ 1 2 3 ; 4 5 6 ; 7 8 9 ]
matrix =
1 2 3
4 5 6
7 8 9
To replace the first row of the matrix, type matrix(1,:)=[-1 -2 -3]
This command replaces the old values [1 2 3] of the first row of the matrix with new values [-1 -2 -3].
>> matrix(1,:)=[-1 -2 -3]
matrix =
-1 -2 -3
4 5 6
7 8 9
Next, type matrix(2,:)=[-4 -5 -6] to replace the values in the second row of the matrix.
>> matrix(2,:)=[-4 -5 -6]
matrix =
-1 -2 -3
-4 -5 -6
7 8 9
Finally, type matrix(3,:)=[-7 -8 -9] to replace the elements in the third row of the matrix.
>> matrix(3,:)=[-7 -8 -9]
matrix =
-1 -2 -3
-4 -5 -6
-7 -8 -9
How to replace a column in the matrix
First, create a 3x3 square matrix.
>> matrix = [ 1 2 3 ; 4 5 6 ; 7 8 9 ]
matrix =
1 2 3
4 5 6
7 8 9
To modify the first column of the matrix, type matrix(:,1)=[-1 -2 -3]
This command changes the values of the first column [1 2 3] of the matrix to other values [-1 -2 -3].
>> matrix(:,1)=[-1 -2 -3]
matrix =
-1 2 3
-2 5 6
-3 8 9
Next, type matrix(:,2)=[-4 -5 -6] to replace the second column of the matrix.
>> matrix(:,2)=[-4 -5 -6]
matrix =
-1 -4 3
-2 -5 6
-3 -6 9
Finally, type matrix(:,3)=[-7 -8 -9] to change the third and final column of the matrix.
>> matrix(:,3)=[-7 -8 -9]
matrix =
-1 -4 -7
-2 -5 -8
-3 -6 -9
In this way, you can change any row or column of a matrix in Matlab.