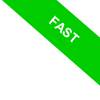
Python's Standard Library
Think of Python's standard library as a built-in toolkit. It's a comprehensive collection of modules and functions that come pre-packaged with Python, thus extending its programming capabilities. No need for additional package installations; it's ready to use out-of-the-box.
This library covers a wide array of functionalities, from mathematical operations and system interactions to file and directory management, text and number manipulation, and even database access. Not to forget its ability to interface with various web services. This extensive range of features is what Python's motto, "Batteries included", stands for.
The standard library's modules are versatile, each serving a unique purpose.
Here are a few examples of modules:
- os
The 'os' module facilitates interaction with the operating system. It's handy for tasks like file reading and writing, file path management, executing system commands, and accessing environment variables. - sys
It presents functions and variables essential for manipulating Python's runtime environment. - re
It enables working with regular expressions, a useful tool for text string searching and manipulation. - math
This module offers mathematical functions for numeric operations like trigonometric calculations, exponential, logarithmic, and other common mathematical functions. - statistics
This module brings mathematical and statistical functions.. - datetime
The 'datetime' module is designed for handling dates and times. - csv
This module grants features for reading and writing data in CSV format, allowing interaction with files and data structured in this common format. - json
It provides functions for reading and writing files in JSON format. - http.client, ftplib, socket
These modules provide functions for working with network protocols such as HTTP, FTP and low-level sockets. - sqlite3
This module offers an interface for working with SQLite databases.
To tap into the power of these modules, simply import them into your Python environment using the import statement, such as 'import math'.
import math
This action loads all the module's functions into memory.
import math
Once a module is imported, you can call any of its functions by writing the module's name followed by a period and then the function's name.
For example, type math.cos(1)
math.cos(1)
This method calculates the cosine of 1.
0.5403023058681398
With this approach, you have at your disposal every function from the module you've imported, streamlining your coding process.