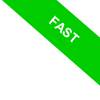
Python's Sequence Repetition
Sequence repetition in Python is an exceptionally practical feature, streamlining and automating repetitive tasks in your code. Whether it's a string, list, tuple, or more, repeating sequences can simplify your projects significantly. Let’s dive into how you can apply this concept across different scenarios.
Note that in Python, using the * operator to repeat a set() is not possible. Sets are unique as they are unordered collections of distinct elements. Repetition, by nature, creates duplicates, conflicting with the set's principle of maintaining uniqueness. This restriction also applies to dictionaries, which are key-value mapping structures requiring unique keys.
String Repetition Simplified
Repeating strings in Python couldn't be simpler. The * operator, coupled with a numeric value, repeats a string as many times as specified. Let’s see it in action.
For example, this command:
repeat = "Python! " * 3
Repeats the string "Python! " threefold.
Check the result with a quick print:
print(repeat)
And there you have it: "Python! " repeated thrice.
Python! Python! Python!
This example illustrates the ease and efficiency of the multiplication operator *
for string repetition in Python.
Repeating Lists with Ease
Similarly, lists can be replicated using the same * operator. Here’s how it works.
Typing this:
list = [1, 2, 3] * 2
Tells Python to duplicate the list [1, 2, 3] twice.
See the result for yourself:
print(list)
Yielding a concatenated list where the original sequence appears twice:
[1, 2, 3, 1, 2, 3]
Advanced Repetition with List Comprehensions
Python’s list comprehensions offer a compact way to create lists. Combine them with sequence repetition to craft more intricate lists. Here’s an example:
Create a list of squares for numbers 1 to 3, then repeat:
squares = [i**2 for i in range(1, 4)] * 2
Display the result:
print(squares)
The initial list [1, 4, 9]
represents squares of 1, 2, and 3. Repeating it, we get:
[1, 4, 9, 1, 4, 9]
Repeating Tuples
Despite their immutable nature, tuples can also be repeated. For example:
Start with a basic tuple:
tuple = ('a', 'b') * 2
Printing it reveals the repetition:
('a', 'b', 'a', 'b')
Each element from the original tuple is duplicated in sequence.
Concatenation and Repetition Combined
Sequence concatenation followed by repetition can lead to powerful combinations. For instance:
Concatenate two lists and then duplicate:
combined = (['a', 1] + ['b', 2]) * 2
Resulting in a list where the combined sequence is repeated:
['a', 1, 'b', 2, 'a', 1, 'b', 2]
A Word of Caution on Mutable Objects
Be mindful when repeating lists containing mutable objects, like other lists. Repetition here creates references to the same object rather than duplicates. Here's an example:
Start with a simple list:
list = [1]
Repeat and nest it:
list_of_lists = [list] * 3
Initially, this appears as three separate lists:
[[1], [1], [1]]
Modify the original list:
list[0] = 2
Reprinting the nested list reveals a surprising change:
[[2], [2], [2]]
This occurs because each nested list references the same original object, not distinct copies.