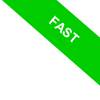
Concatenating Sequences in Python
Python's sequences, such as lists, tuples, strings, etc., are known for their flexibility, particularly in concatenation.
What Exactly is Concatenation? Simply put, concatenation is the process of combining two or more sequences of elements (like strings, lists, tuples) into a single, cohesive sequence. Each element of the first sequence is followed by the elements of the subsequent sequences, creating a new, unified sequence. Take for instance two strings: "ABCD" and "EFG". Concatenating them yields "ABCDEFG", merging the original strings into a single, fluid entity.
Let’s delve into the various ways these sequences can be joined together.
String Concatenation
In Python, string concatenation is straightforward, achieved using the '+' operator.
Here's an illustration. Start by creating two strings:
str1 = "Hello"
str2 = "World"
Combine these strings, assigning the result to a new variable.
concatenated_str = str1 + " " + str2
Now, reveal the new variable's content.
print(concatenated_str)
The resulting variable is a unified string, a blend of the initial pair.
"Hello World"
For extensive strings or frequent concatenations, methods like str.join() are more efficient.
List Concatenation
Similarly, lists can be concatenated using the '+' operator.
Consider the following example. Create two lists:
list1 = [1, 2, 3]
list2 = [4, 5, 6]
Concatenate these lists into a third entity.
concatenated_list = list1 + list2
Examine the merged list's contents.
print(concatenated_list)
This list now seamlessly combines elements from both the first and second lists.
[1, 2, 3, 4, 5, 6]
Consider list comprehension for larger lists.
Tuple Concatenation
Despite their immutable nature, tuples can be concatenated in a manner akin to lists.
For instance, create two tuples:
tuple1 = (1, 2, 3)
tuple2 = (4, 5, 6)
Merge these tuples and store the result in a new variable.
concatenated_tuple = tuple1 + tuple2
Display the contents of this new variable.
print(concatenated_tuple)
The outcome is a six-element tuple, a fusion of the two originals.
(1, 2, 3, 4, 5, 6)
Remember, sequence concatenation requires the same type of sequences. For example, a list cannot be directly concatenated with a tuple.
Array Concatenation
Arrays in Python can be concatenated using specialized methods like 'extend'.
Begin by importing the array module:
from array import array
Create two arrays:
array1 = array('i', [1, 2, 3])
array2 = array('i', [4, 5, 6])
Use the extend() method to merge them.
array1.extend(array2)
Review the content of the extended array.
print(array1)
The first array now encompasses elements from the second.
array('i', [1, 2, 3, 4, 5, 6])
Keep in mind that lists and arrays are mutable, while strings and tuples are not. Thus, every concatenation of strings or tuples results in a new object.
This guide offers a succinct yet comprehensive overview of sequence concatenation in Python, essential for any budding or experienced programmer.