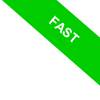
List Comprehension in Python
In Python, list comprehension is an elegant and powerful tool that facilitates the creation and manipulation of lists. By providing a more succinct syntax, it not only enhances readability but also adds a layer of conciseness to your code.
Consider the following example.
Suppose you want to create a list of numbers from 1 to 5 and store it in the variable "numbers".
numbers = [1, 2, 3, 4, 5]
You can then define a second list called "squares" using list comprehension. This list will contain the squares of the elements found in "numbers".
squares = [n**2 for n in numbers]
This list comprehension is read as: "for each element n in numbers, calculate n squared."
Printing the "squares" list gives you.
print(squares)
Here, `squares` is a newly created list consisting of the squares of every element in `numbers`.
[1, 4, 9, 16, 25]
List comprehension also allows for the application of specific conditions.
For instance, you could create a list of squares solely for the even numbers within `numbers`.
even_squares = [n**2 for n in numbers if n % 2 == 0]
This translates to: "for each element n in numbers, calculate n squared, but only if n is even."
The above expression iterates over the elements in "numbers," considering only those that meet the condition of being even – that is, numbers leaving no remainder after division by two.
Printing the "even_squares" list:
print(even_squares)
In this instance, the list consists exclusively of the squares of the numbers 2 and 4.
[4, 16]
Though these examples may appear simple, they effectively illustrate the functionality of list comprehension in Python.
By embracing this feature, you can write code that's not only more efficient but also aesthetically pleasing.
It's a technique that beautifully encapsulates Python's philosophy of writing clear, logical code, and is a must-know for anyone seeking to deepen their understanding of this versatile language.