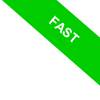
Python Sequences
Sequences in Python are key structures for data management and organization. Think of them as versatile containers that systematically store various elements, either in a numeric sequence or based on the order they were added.
These iterable objects in Python enable you to neatly arrange collections of data in a predetermined order. Elements in a sequence are sequentially indexed from left to right. This means every element is assigned an index, starting with 0 for the first element. This indexing serves as a pivotal key, facilitating the search, retrieval, or alteration of any single element within the sequence without impacting the rest.
Diverse types of sequences exist in Python, each with its own set of features and capabilities. The most commonly used are:
- Lists
These mutable sequences allow element modifications. They are versatile, capable of containing mixed data types. For example, the list lista = [1, "two", 3.0] combines an integer, a string, and a floating-point number.lista = [1, "two", 3.0]
- Tuples
Similar to lists, but immutable. Once created, their elements remain constant, making them ideal for data integrity. For instance, the tuple tupla = (1, 2, 3) consists of three elements.tupla = (1, 2, 3)
- Strings
In Python, strings are sequences of characters. Each character in a string corresponds to a position in the sequence, identified by an index. For example, the string stringa="Nigiara" is a sequence of 7 characters.stringa = "Nigiara"
- Range
This sequence type is primarily used for generating numerical series, often utilized in loops for a set number of iterations. For instance, range(5) creates a sequence from 0 to 4.range(5)
Each sequence type in Python supports a variety of operations such as indexing, slicing, adding, and removing elements, among others.
For example, consider creating a list with five elements:
>> lista = ['one', 'two', 'three', 'four', 'five']
To determine the count of elements in a sequence, the len() function is used. In this example, the list contains five elements.
>> len(lista)
5
Accessing a specific element in a sequence is straightforward – simply use its index within square brackets.
For instance, retrieve the first element of the list with lista[0].
>> lista[0]
one
Remember, Python indexing starts at zero, so the second element is accessed with index 1.
>> lista[1]
two
Slicing is a powerful feature that allows you to extract a subsequence from a sequence, defined by a start and end index. For example, lista[1:3] yields the second and third elements of the list.
>> lista[1:3]
['two', 'three']
Slicing can also incorporate negative indices. In this context, -1 refers to the last element, viewing the sequence in reverse.
>> lista[-1]
five
Similarly, -2 would refer to the second-last element, and so on.
>> lista[-2]
four
Python sequences are inherently iterable, making them highly suitable for performing operations on each element, especially when combined with loops like for or while.
Empty Sequences in Python
In Python, empty sequences are consistently evaluated as False, while sequences with any content are considered True. This fundamental concept applies across several data structures, including strings, lists, and tuples.
Take, for example, an empty string (''). In a Boolean context, this is interpreted as False.
bool('')
False
On the other hand, a string that contains even a single character, such as a space (' '), is evaluated as True.
bool('abc')
True
The same logic extends to lists. An empty list ([]) equates to False.
bool([])
False
Conversely, a list with at least one item, even if it's an empty string (['']), is deemed True.
bool(['abc', 'def'])
True
Tuples follow suit: an empty tuple (()) is False.
bool(())
False
However, a tuple containing at least one element, even an empty one (('',)), is regarded as True.
bool(('abc','def'))
True
This nuanced approach to evaluating truth and falsehood in Python sequences is essential for effective programming.
In essence, any sequence holding content, irrespective of its nature, is evaluated as True, while a total lack of content results in a False evaluation.