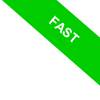
Lists in Python
In this Python course lesson, I'll explain how to use a list.
What are lists? They're a type of data structure that allows you to store an ordered series of values. Each value is associated with a position in the list. They belong to the sequence class, like strings. However, unlike strings, lists can contain objects of any type: numbers, strings, boolean values, and other objects as elements.
For example, here's a list made up of three different types of elements, a string, a number, and a boolean value.
>>> list = ['abc', 127, True]
To create a list in Python, you need to indicate the elements of the list inside square brackets, separated by commas.
>>> list = [element1, element2, ...]
One of the main characteristics of lists is their flexibility. At any time, you can directly access each individual element, add, remove, or modify the elements of a list.
An Example of a List
Let's take a practical example.
Here's a list composed of four alphanumeric values:
>>> fruit = ['apple', 'banana', 'pear', 'orange']
In this example, the list is associated with a variable called "fruit".
The list contains four string elements: "apple", "banana", "pear", and "orange".
Note. Since they are alphanumeric values, you must indicate each element between double quotes.
Each element of the list is associated with an index that indicates its position in the list.
- The first element "apple" is associated with index 0.
- The second element "banana" is associated with index 1.
- The third element "pear" is associated with index 2.
- The fourth element "orange" is associated with index 3.
Note. In Python, list indices start from 0, which means that the first element of the list always has an index equal to 0, the second element has an index equal to 1, and so on.
Thanks to the indices, you can directly access each individual element of the list.
Creating Lists in Python
Python offers a variety of methods for list creation.
One straightforward approach involves literally defining a list: simply encase your elements in square brackets, separating each with a comma.
>>> my_list = ['abc', 127, True]
An alternative method employs the list class.
>>> my_list = list(('abc', 127, True))
Here, you're required to present the elements within an iterable ('abc', 127, True) and then invoke it as an argument of the list class.
When the iterable is a string, Python interprets each character as a separate element.
>>> my_list = list('abc')
This method results in a list comprising three elements.
>>> print(my_list)
['a', 'b', 'c']
List comprehension offers a more dynamic way to define lists. This approach is particularly effective when dealing with numerous elements.
>>> my_list = [i for i in range(10)]
This simple line of code generates a list of integers, ranging from 0 to 9.
>>> print(my_list)
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
For more complex structures, such as matrices, nesting multiple list comprehensions is highly effective.
Consider this example, where two list comprehensions are used to construct a 3x3 matrix.
>>> matrix = [[3 * i + j + 1 for j in range(3)] for i in range(3)]
This technique yields a matrix with three rows and three columns, sequentially numbered from 1 to 9.
>>> print(matrix)
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
In summary, list comprehension is not only versatile but also tends to be more efficient than traditional methods like append().
How to Access List Elements
To access the elements of a list, use the indexing operator [n].
For example, you have a list with 4 elements in the variable "fruit".
>>> fruit = ['apple', 'banana', 'pear', 'orange']
Type fruit[0] to access the first element of the "fruit" list that you just created.
>>> fruit[0]
Python accesses the "fruit" list, reads and returns the value in the first position (index zero).
In this case, the first element is the value "apple".
apple
Now type the command fruit[1]
>>> fruit[1]
Python accesses the "fruit" list, reads and returns the second value of the list (index one).
In this case, the second element is the value "banana".
banana
Similarly, you can access all the other elements of the list.
How to Add Elements to a List
To add an element to the end of the list, use the append method:
list_name.append(new_element)
It's a predefined method of lists in Python that adds the element specified inside the parentheses to the end of the list.
Let me give you a practical example.
You have a list with 4 elements in the variable "fruit".
>>> fruit = ['apple', 'banana', 'pear', 'orange']
Type fruit.append('lemon') to add a new element called "lemon" to the "fruit" list.
>>> fruit.append('lemon')
Then display the contents of the "fruit" variable.
Now there are five values in the list. The new element "lemon" has been added to the end of the list.
>>> fruit
['apple', 'banana', 'pear', 'orange', 'lemon']
In Python, you can use the len() function to count the number of elements in a list.
To do so, simply type len(fruit) on the command line and press enter.
>>> len(fruit)
The function returns the length of the "fruit" list.
For instance, if the list contains five elements, the output will be 5.
5
How to remove elements from a list
There are different ways to remove elements from a list in Python.
Let's take a look at some examples:
1] The remove() method
This method allows you to remove the first element with a specific value from the list.
For example, let's say you have a list called "fruit" consisting of five elements, including "apple", "banana", "pear", "orange", and "lemon".
>>> frutta
['apple', 'banana', 'pear', 'orange', 'lemon']
To remove the element "banana" from the list, type fruit.remove('banana') on the command line.
>>> fruit.remove('banana')
After executing this command, the element "banana" will be removed from the "fruit" list.
>>> fruit
['apple', 'pear', 'orange', 'lemon']
2] The pop() method
This method allows you to remove an element from the list based on its index.
For example, if you have a list consisting of four elements, including "apple", "pear", "orange", and "lemon".
>>> frutta
['apple', 'pear', 'orange', 'lemon']
You can remove the third element from the list by typing fruit.pop(2) on the command line.
>>> fruit.pop(2)
In this case, the pop() method removes the element with index 2 (i.e., the third element), which is "orange".
>>> frutta
['apple', 'pear', 'lemon']
3] The del() function
This function removes an element from the list based on its index.
For instance, if you have a list consisting of three elements, including "apple", "pear", and "lemon".
>>> frutta
['apple', 'pear', 'lemon']
You can remove the first element from the list by typing del fruit[0] on the command line.
del fruit[0]
This will remove the element "apple" from the list.
>>> fruita
['pear', 'lemon']
By using these methods and functions, you can easily modify your Python lists according to your needs.