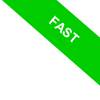
Python's Augmented Assignment Operators
Augmented assignment operators serve as a shortcut in Python, enhancing code brevity and clarity by avoiding redundant variable names in assignment statements.
Consider this: instead of the verbose a = a + 2, you can succinctly write a += 2. This simple example neatly showcases the efficiency of augmented assignments in Python programming.
Python features two primary augmented assignment operators for addition and multiplication:
The += Operator
The += operator simplifies addition assignments.
It adds the right-hand value to the left-hand variable and reassigns the sum back to that variable. For instance:
Begin by assigning the value 5 to a variable:
a=5
Then, elegantly add 2 using the += operator:
a += 2
This is functionally identical to a = a + 2.
Display the result:
print(a)
The outcome? A neat 7.
7
The *= Operator
The *= operator is your go-to for multiplication assignments.
It multiplies the variable by the right-hand value and reassigns the product back to that variable.
As an example, assign a value to variable b:
b = 3
Apply the *= operator like so:
b *= 4
Effectively, this is the same as b = b * 4.
It multiplies the initial value in b by 4.
Show the result:
print(b)
The result? A solid 12, quadrupling the initial value of 3 in b.
12
To sum up, augmented assignments enable the same operations as + and * but in a more streamlined fashion.
Augmented Assignments and Sequences: Lists, Tuples, Strings
Augmented assignments are also versatile with sequences such as strings, lists, and tuples, offering interesting functionalities for each.
Lists
With lists, the += operator adds elements seamlessly to the end.
Create a list with three elements:
lista = [1, 2, 3]
Then extend it effortlessly using the += operator:
lista += [4, 5]
Resulting in:
[1, 2, 3, 4, 5]
The *= operator duplicates and concatenates the list a specified number of times.
Let's redefine the list with three elements:
lista = [1, 2, 3]
Now double it:
lista *= 2
Voilà, a list with six elements, repeating the sequence twice.
[1, 2, 3, 1, 2, 3]
Strings
The += operator appends strings with elegance.
Start with a string:
stringa = "Hello"
Then concatenate another string:
stringa += " World"
Yielding a smoothly combined phrase:
"Hello World"
The *= operator repeats a string a given number of times.
Define a string:
stringa = "abc"
Triple it effortlessly:
stringa *= 3
Resulting in a thrice-repeated sequence:
"abcabcabc"
Note: Using *= on strings or lists for creating large datasets can be memory and performance intensive.
Tuples
The += operator is incompatible with tuples due to their immutable nature.
However, the *= operator allows for repetition of tuple elements.
The *= operator multiplies and concatenates tuple elements.
For example, create a tuple with two elements:
tupla = (1, 2)
Then, repeat it thrice:
tupla *= 3
The tuple evolves into a six-element structure.
(1, 2, 1, 2, 1, 2)
In conclusion, Python's augmented assignments offer a sophisticated way to refine variable and sequence manipulation, enhancing code efficiency and readability.