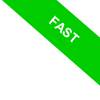
Unpacking in Python
Python's unpacking feature is an efficient tool that permits the assignment of values from a sequence (such as a tuple, list, etc.) to multiple variables, all in one go.
Let's consider a tangible example to comprehend it better.
Initially, construct a tuple comprising three elements.
tuple = (1, 2, 3)
Subsequently, proceed to unpack the tuple as follows:
a, b, c = tuple
When it comes to unpacking, it's critical to match the number of variables with the quantity of elements in the sequence. Failing to do so will result in Python generating a 'too many values to unpack' error. However, there's no need for concern - Python facilitates partial unpacking to circumvent this issue. We'll touch on this towards the conclusion of this tutorial.
Here's what happens when Python gets to work:
The first tuple value, which is 1, gets assigned to the variable 'a'
print(a)
1
The second tuple value, 2, is allocated to the variable 'b'
print(b)
2
And the third tuple value, 3, is designated to the variable 'c'
print(c)
3
But it doesn't stop there - unpacking is equally effective with lists and strings.
For instance, you can create a list:
list = [1, 2, 3]
And promptly unpack this list into three separate variables.
a, b, c = list
Likewise, for a string, you'd first create one:
string = "xyz"
And then proceed to unpack each string character into three variables.
a, b, c = string
Partial Unpacking in Python
Python also caters to partial unpacking, achieved by prefixing a variable with an asterisk "*".
The asterisk * is utilized to signify that the remaining variable should accumulate any excess values during the unpacking process.
Consider the following list:
list = [1, 2, 3, 4, 5]
Now, assign the initial two elements to the variables 'a' and 'b' and the residual elements to the variable 'c'.
a, b, *c = list
Here's what unfolds:
The first list element gets assigned to variable 'a'.
print(a)
1
The second list element is allocated to variable 'b'.
print(b)
2
Variable 'c' is assigned all remaining list elements, starting from the third element.
print(c)
[3, 4, 5]
In this example, 'a' and 'b' secure the first two elements, while 'c' incorporates the remaining tuple elements as a list.
It's important to note that while variables 'a' and 'b' each contain a single numerical value, variable 'c' is a list - a data structure housing a sequence of values - as it accommodates multiple values.