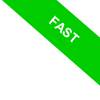
Tuples in Python
In Python, tuples are sequences of immutable objects. This means that once you've created a tuple, it can't be modified.
So what sets lists apart from tuples? Lists in Python are mutable data structures enclosed by square brackets, perfect for dynamic collections of elements that evolve over time. Tuples, on the other hand, are immutable data structures defined within round brackets. They're used to group information that won't change during the program execution.
Creating a Tuple
To craft a tuple, simply list the elements you want, separated by commas, and enclose them in parentheses.
my_tuple = ('element1', 'element2', 'element3')
Interestingly, tuples can also be formed without the parentheses.
my_tuple = 'element1', 'element2', 'element3'
Alternatively, the tuple() class offers another approach.
my_tuple = tuple('element1', 'element2', 'element3')
This class is particularly versatile as it can take any iterable, like a list, as an argument.
list = ['element1', 'element2', 'element3']
my_tuple = tuple(list)
No matter which method you choose, the end result remains a tuple.
A word of caution when defining a single-element tuple: remember to include a comma after the element. Without it, Python will treat it as a solitary value rather than a tuple. Thus, the correct syntax for a single-element tuple is:
my_tuple = ('single_element',)
For tuples with two or more elements, the parentheses are optional.my_tuple = 'element1', 'element2', 'element3'
Let's look at a real-world example.
Create a tuple containing the names of the seasons:
seasons = ('spring', 'summer', 'fall', 'winter')
Next, let's display the tuple:
print(seasons)
Python will then show all the elements within the tuple.
('spring', 'summer', 'fall', 'winter')
For an empty tuple, simply use the tuple class without any arguments inside the parentheses.
my_tuple = tuple()
To confirm that your creation is a tuple, the type() function is handy.
print(type(my_tuple))
If it's a tuple, the function will return <class 'tuple'>.
<class 'tuple'>
Accessing Tuple Elements
Accessing elements of a tuple can be achieved through indexing. To do this, indicate the index of the desired element in square brackets immediately following the tuple name.
my_tuple[index]
Remember, in Python, indexing starts at zero. So, the first element of the tuple is at index zero, the second at index one, and so forth.
For instance, create a tuple in the variable "seasons".
seasons = ('spring', 'summer', 'autumn', 'winter')
Then print the first element of the tuple by indicating seasons[0] in the print() statement.
print(seasons[0])
Python accesses the element at index zero - the first element of the tuple - and displays it on the screen.
spring
To access the second element, write seasons[1] in the print() statement.
print(seasons[1])
Python reads and returns the element at index one, i.e., the second element of the tuple.
summer
This is how you can access each individual element of the tuple.
Tuple Immutability
Tuples are immutable, which means you can't alter, add, or remove elements from a tuple once it has been defined.
Consider the previous tuple as an example.
seasons = ('spring', 'summer', 'autumn', 'winter')
This tuple consists of four elements.
Now, try to change the first element of the tuple.
seasons[0] = 'test'
When you attempt to modify a tuple, Python throws an error message.
TypeError: 'tuple' object does not support item assignment
Iterating Over a Tuple
You can iterate over the elements of a tuple much like you would with a list.
For example, create the tuple
seasons = ('spring', 'summer', 'autumn', 'winter')
Then iterate over the list elements using a for loop.
- for element in seasons:
- print(element)
In each iteration, Python accesses a list element and prints it to the screen.
spring
summer
autumn
winter
Tuple Functions and Methods
Python has a variety of useful functions and methods for working with tuples.
For example, create a tuple.
my_tuple = (1, 2, 3, 2, 4, 4, 4)
Then use the len() function to find the number of elements.
print(len(my_tuple))
The tuple has 7 elements.
7
The max() function helps you identify the maximum value.
print(max(my_tuple))
The maximum value in the tuple is 4.
4
The min() function identifies the minimum value in the tuple.
print(min(my_tuple))
The minimum value in the tuple is 1.
1
The count() method counts the number of times an element appears in the tuple.
print(my_tuple.count(4))
Prints 3, the number of times 4 appears in the tuple.
3
The index() method locates the item with a specified index number.
print(my_tuple.index(2))
In this case, at index 1 in the tuple is stored the value 2.
1
Remember, the application of tuples is broad and you'll frequently encounter them in your coding journey.
Understanding tuples is a fundamental aspect when working with Python.