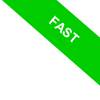
Python's range() Function
The range() function in Python is a real workhorse. It generates an iterable object, spitting out a tidy sequence of numbers.
range(start, stop, step)
These three parameters have different roles in the script, with only "stop" being mandatory.
- Start
Think of it as the kick-off point for your number sequence. It starts at zero if you don't specify otherwise. - Stop
This is the finishing line of your number sequence - it's non-negotiable. - Step
It's the skip or hop between numbers. If you leave it out, it defaults to a simple one-step jump.
In essence, our trusty range() function gifts us with a sequence of numbers.
This gem is often spotted in "for" loops, where it works hard repeating a block of code a set number of times or taking us on a journey through a sequence of numbers.
You can call on the range() function in three different ways:
range(stop)
Here, you'll get a sequence of numbers starting from 0 and ending just before the stop value.
range(start, stop)
This variation gives you a sequence starting at your chosen start value and finishing just shy of the stop value.
range(start, stop, step)
In this version, you get a sequence that starts at your chosen value, takes the step into account for each hop, and stops before reaching the stop value.
Here's a real-world example to demonstrate the range function's usefulness:
- for i in range(5):
- print(i)
This little snippet will neatly print out the numbers from 0 to 4.
0
1
2
3
4
You'll notice that the stop value of 5 is intentionally omitted from the sequence.
When you run the range function, it's worth noting that it churns out a "range" type object.
For instance, if you want to generate a sequence of odd numbers from 1 to 9, you'd play with the parameters like so: start=1, stop=10, and step=2.
>>> x=range(1,10,2)
To check what kind of object you're dealing with, you can use the type() function.
>>> type(x)
This will confirm that you've got an iterable object of the "range" variety.
<class 'range'>
To reveal the contents of a "range" object, it needs to be morphed into a list or a tuple.
>>> list(x)
Now you'll see the sequence generated by the range function, specifically, the odd numbers from 1 to 9.
[1, 3, 5, 7, 9]
A key takeaway to remember is that while range objects are adept at handling common sequence operations, they draw the line at concatenation and repetition. These would just complicate matters when it comes to indexing.