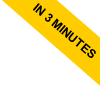
Predefined functions in Scilab
This page offers a comprehensive overview of the primary commands and functions available in Scilab.
In the first section, you'll encounter essential, frequently used base commands. The second section introduces a collection of other useful commands and functions, particularly pertinent in specific circumstances.
Basic Commands
Here are some commonly used base functions and commands in Scilab:
- clc
Clears the command window, removing all displayed commands and results. - clear
Wipes all variables from the workspace. For instance, clear x eliminates variable x from memory, whereas clear alone removes all variables. - disp
Displays the value of a variable or a text message. For instance, disp(x) prints the value of variable x, while disp('Hello, Scilab!') prints the message "Hello, Scilab!". - help
Provides syntax and usage information about a specific function. For example, help plot supplies information on using the plot() function. - length
Returns the number of elements in a vector or the largest dimension of a matrix. For instance, length(v) yields the number of elements in vector v. - load
Loads variables from a file. For instance, load('myfile.sce') pulls variables from a file named myfile.sce. - pause
Halts program execution for a given number of seconds. For instance, pause(5) interrupts execution for 5 seconds. - save
Stores the current variables in a file. For instance, save('myfile.sce') saves current variables in a file named myfile.sce. - size
Returns the number of rows and columns in a matrix or a vector. For instance, size(A) yields the number of rows and columns in matrix A. - who
Lists the variables currently in the workspace. - whos
Gives a detailed list of variables in the workspace, including their size and data type.
Other Commands
Here's a roster of other usable commands and functions in Scilab:
- a2s
Converts an array into a string. - abs
Calculates the absolute value of a number or each element of an array. - acos
Computes the arccosine of a number or each element of an array. - acosh
Calculates the hyperbolic arccosine of a number or each element of an array. - addf
Adds two functions together. - adj2sp
Transforms an adjacency matrix into a sparse matrix. - affich
Displays a message in the command bar. - aligntol
Aligns a matrix to a specified point. - alog10
Calculates the base 10 logarithm of a number or each element of an array. - and
Computes the logical AND between two arrays. - argn
Returns the number of input or output arguments for a function. - asin
Computes the arcsine of a number. - asinh
Computes the hyperbolic arcsine of a number or each element of an array. - atan
Computes the arcsine of a number. - atanh
Computes the hyperbolic arctangent of a number or each element of an array. - balanc
Balances a matrix. - band
Creates a matrix with a band. - bar
Generates a bar chart. - base2dec
Converts a string of numbers in base n to a decimal number. - bernoulli
Generates a number from a Bernoulli distribution. - besselh, besseli, besselj, besselk, bessely
Bessel functions. - beta
Beta function. - bin2dec
Converts a binary number to decimal - binomial
Generates a number from a binomial distribution. - bitand, bitor, bitxor, bitcmp, bitget, bitset, bitshift
Bitwise operations. - blackman
Blackman window. - blockdiag
Constructs a block diagonal matrix. - boolean
Creates a Boolean value. - cat
Concatenates matrices or vectors along a specific dimension. - cd
Changes the current working directory. - ceil
Rounds a number up to the next largest integer. - chdir
Changes the current working directory. - clc
Clears the command prompt window. - clear
Wipes all variables from the workspace. - clf
Clears the current figure. - close
Closes a graphic window. - colormap
Changes or retrieves the current color map. - complex
Generates a complex number from a real and an imaginary part. - cond
Computes the condition number of a matrix. - conj
Calculates the complex conjugate of a number. - continue
Skips the remainder of the current iteration in a loop and moves to the next iteration. - cos
Calculates the cosine of an angle. - cosh
Computes the hyperbolic cosine of a number. - csvRead
Reads data from a CSV file. - csvWrite
Writes data into a CSV file. - cumprod
Computes the cumulative product of the elements of a vector or a matrix. - cumsum
Calculates the cumulative sum of the elements of a vector or a matrix. - datenum
Converts a date and time to a serial number. - datevec
Converts a date serial number into a vector of date components. - dbclear
Removes breakpoints. - dbcont
Resumes debug execution. - dbquit
Exits from debug mode. - dbstack
Displays the position of the current function in the debug file. - dbstatus
Displays all breakpoints. - dbstop
Sets a breakpoint. - dec2bin
Converts a decimal number to binary. - dec2oct
Converts a decimal number to octal. - dec2hex
Converts a decimal number to hexadecimal. - det
Computes the determinant of a matrix. For example, det(A) computes the determinant of matrix A. - diag
Returns a diagonal matrix from a vector, or yields a vector containing the diagonal elements of a matrix. For example, diag([1, 2, 3]) returns a 3x3 diagonal matrix with 1, 2, and 3 on the diagonal. - diff
Calculates the difference between successive elements of a vector. - disp
Displays the value of a variable or a text message. - dlmread
Reads data from a delimited file. - dlmwrite
Writes data into a delimited file. - dot
Computes the dot product of two vectors. - %e
This constant returns Euler's number. - eigs
Computes some eigenvalues and eigenvectors of a matrix. - eig
Computes the eigenvalues and eigenvectors of a matrix. For example, [V, D] = eig(A) computes the eigenvalues (D) and eigenvectors (V) of matrix A. - eps
Returns machine precision (minimum detectable difference between two floating-point numbers). - erf
Calculates the error function. - erfc
Calculates the complementary error function. - erfinv
Calculates the inverse of the error function. - erfcinv
Calculates the inverse of the complementary error function. - evstr
Evaluates a string as a Scilab command. - eval
Evaluates an expression or a string. - exp
Computes the exponential of each element in a matrix. - expm
Calculates the exponential of a matrix. - eye
Creates an identity matrix of a specified size. For instance, eye(3,3) creates a 3x3 identity matrix. - exec
Executes a Scilab script file. - exists
Checks for the existence of a variable. - exit
Exits from Scilab. - %f
Represents the Boolean value "False". - factor
Computes the factorization and returns the list of prime factors for a given number. - factorial
Calculates the factorial n! of a non-negative integer. - feval
Executes a function specified by a string or a function handle. - find
Returns the indices of the elements in a vector or a matrix that satisfy a certain condition. For instance, find(A > 0) returns the indices of the elements in A that are greater than 0. - format
Set the number of digits in the representation of numbers. - fft
Performs the Fast Fourier Transform. - fftw
Functions for the Fourier Transform. - fscanf
Reads data from a file based on a specified format. - fseek
Moves the position pointer within a file. - fsolve
Solves nonlinear equations. - full
Converts a sparse matrix to a full matrix. - fprintf
Writes data to a file based on a specified format. - fread
Reads binary data from a file. - fwrite
Writes binary data to a file. - gca
Returns the handle to the current entity ("get current axes"). - gcf
Returns the handle to the current graphical window ("get current figure"). - get
Retrieves the value of a specific property of a graphic handle. - getcolor
Opens a dialogue box to select a color. - getf
Retrieves the number associated with a figure from a handle or figure name. - getdate
Returns the current time and date as a vector. - grayplot
Creates a grid plot. - grid
Adds a grid to a current plot. - gradient
Calculates the gradient of a matrix. - grand
Generates random numbers from various distributions. - hav
Calculates the haversine function. - halt
Interrupts the execution of the code. - hankel
Creates a Hankel matrix. - hex2dec
Converts a hexadecimal number to decimal. - hilb
Creates a Hilbert matrix. - hist3d
Creates a 3D histogram. - histplot
Creates a bar plot. - histm
Calculates the histogram of a matrix. - h2norm
Calculates the H2 norm. - help
Provides help on a topic or function. - hess
Calculates the Hessenberg form of a matrix. - %i
Used to represent the imaginary unit, equivalent to sqrt(-1). - %inf
Infinity symbol - ifft
Performs the Inverse Fourier Transform. - imag
Returns the imaginary part of a complex number. - import
Imports data from files in various formats, including CSV and Excel. - int
Converts a floating-point number to an integer. - int8
Converts a number to an 8-bits integer - int16
Converts a number to a 16-bits integer. - int32
Converts a number to a 32-bits integer. - int64
Converts a number to a 64-bits integer. - integrate
Performs numerical integration of a function. - interp
Performs data interpolation. - inv
Calculates the inverse of a matrix. For example, inv(A) calculates the inverse of the matrix A. - isreal
Checks whether a number is real (that is, if it does not have an imaginary part). - issquare
Checks if a matrix is square. - isvector
Checks if a matrix is a vector. - isinf
Checks if a number is infinite. - isnan
Checks if a number is not a number (NaN). - isdef
Checks if a variable or function is defined. - isempty
Checks if a matrix is empty. - jacf
This function calculates the Jacobian approximation of a function. - jmat
This function returns the Pauli matrix or the J matrix of a quaternion depending on the given arguments. - join
This function joins two or more matrices or vectors along a specific dimension. - jordan
This function calculates the Jordan normal form of a matrix. - jroots
This function calculates the roots of a polynomial with complex coefficients. - %j
This is a predefined constant in Scilab representing the imaginary unit (equivalent to %i in Scilab). - kron
Returns the Kronecker product of two matrices. Usage example: kron(A, B). - kmeans
Performs data clustering using the k-means algorithm. Usage example: kmeans(X, k). - keyboard
Interrupts the execution of a function or a script and gives control to the user. Usage example: keyboard. - kill
Terminates an external process. Usage example: kill(pid). - kfwrite
Writes data in Kelvin format to a file. Usage example: kfwrite('filename', data). - linspace
Generates a linear sequence of points between two limits. For instance, linspace(0, 1, 11) generates 11 evenly spaced points between 0 and 1. - log
Calculates the natural logarithm. - log10
Calculates the base 10 logarithm. - log1p
Calculates the natural logarithm of 1 plus a number. - log2
Calculates the base 2 logarithm. - logspace
Generates a logarithmic sequence of points between two limits. For instance, logspace(0, 1, 11) generates 11 evenly spaced points on a logarithmic scale between 100 and 101. - length
Returns the number of elements in a vector or the maximum dimension of a matrix. - load
Loads variables from a file. - lu
Performs the LU factorization of a matrix. - lqr
Calculates the optimal linear quadratic regulator for a control system. - m2sci
Converts MATLAB or Octave code into Scilab code. - m2scideclare
Declaration for MATLAB to Scilab conversion. - m2scideclareglobal
Declaration for MATLAB to Scilab conversion. - m2scideclarevar
Declaration for MATLAB to Scilab conversion. - m2scideclareview
Declaration for MATLAB to Scilab conversion. - madd
Adds a matrix to another. - mag2db
Converts magnitude to decibel. - makematrix
Creates a matrix. - makelist
Creates a list. - manova
Performs multivariate analysis of variance (MANOVA). - map
Maps a function to each element of a matrix or a list. - matfile_close
Closes a MATLAB matrix file. - matfile_listvar
Lists the variables in a MATLAB matrix file. - matfile_open
Opens a MATLAB matrix file. - matfile_varreadnext
Reads the next variable from a MATLAB matrix file. - max
Finds the maximum value. - mean
Calculates the arithmetic mean. - median
Calculates the median. - menu
Creates an interactive menu. - meshgrid
Creates a 2D coordinate grid used for evaluating functions over a grid. For example, [X, Y] = meshgrid(-10:10, -10:10) generates X and Y matrices of coordinates on a 21x21 grid. - min
Finds the minimum value. - mod
Calculates the modulus (remainder of the division). - nearfloat
This function returns the nearest floating values. - ndims
This function returns the number of dimensions of an array. - newfun
This function allows defining new functions in Scilab. - newlist
This function creates a new list. - newim
This function creates an empty image. - neldermead
This function implements the Nelder-Mead optimization algorithm. - nchoosek
This function calculates the binomial coefficient. - neigh
This function returns the matrix of neighbors of a graph. - nextpow2
This function returns the exponent for the next power of two. - norm
This function calculates the norm of a vector or a matrix. - num2str
Converts a number into a string. For example, num2str(123) returns the string '123'. - oct2dec
Converts a octal number to decimal - ones
Crea una matrice o un vettore di uno. Ad esempio, ones(2, 2) restituisce una matrice 2x2 piena di uno. - or
A logical operator that returns true if at least one of the operands is true. - ode
Used to solve ordinary differential equations. - optim
Implements various optimization methods. - orderfields
Sorts or reorders the fields of a structure. - opengl
Controls the use of OpenGL in Scilab. - output
Configures the output of plots. - orth
Returns an orthonormal basis for the rank of a matrix. - oldmarkstyle
Changes the marking style for previous versions. - oldstyle
Changes the figure building style for previous versions. - %pi
This constant returns the value of pi ( π ) - pade
Calculates the Padé approximation of a function. - pause
Pauses the execution of a script for a specific number of seconds. - plot
Creates a 2D plot of data. - plot2d
Another function for creating 2D plots. - plot3d
Creates a 3D plot of data. - poly
Creates a polynomial from its coefficients or its roots. For example, poly([1, 2, 3], 'x') creates the polynomial x3 - 6x2 + 11x - 6. - print
Prints the contents of a variable or an expression. - printf
Another print function that allows you to format the output. - prod
Calculates the product of the elements of a vector or a matrix. - pwd
Returns the path of the current working directory. - rand
Generates a matrix of random numbers between 0 and 1. For example, rand(3,3) creates a 3x3 matrix of random numbers. - roots
Finds the roots of a polynomial. For example, roots([1, -6, 11, -6]) finds the roots of the polynomial x3 - 6x2 + 11x - 6. - plot
Creates a 2D plot of input data. For example, plot(x,y) creates a plot of x values against y. - q2e
Converts a quaternion into a Euclidean rotation matrix. - q2r
Converts a quaternion into a rotation matrix. - qchol
Performs a Cholesky decomposition of positive definite matrix subject to semi-definiteness. - qderiv
Calculates the derivative quotient of two vectors. - qeig
Calculates eigenvalues and eigenvectors of a symmetric/Hermitian matrix. - qevf
Calculates the quasi-triangular Schur decomposition of a matrix. - qfft
Calculates the Fast Fourier Transform. - qifft
Calculates the Fast Inverse Fourier Transform. - qp
Solves quadratic programming problems. - qr
Calculates the QR decomposition of a matrix. - qrupdate
Updates the QR decomposition of a matrix. - rand
Generates uniformly distributed random numbers between 0 and 1. - randn
Generates normally distributed random numbers. - real
Returns the real part of a complex number. - roots
Calculates the roots of a polynomial. - rank
Determines the rank of a matrix. - reshape
Changes the dimensions of a matrix without altering its data. - round
Rounds a number to the nearest integer. - rem
Calculates the remainder after division, equivalent to the modulo operator. - repmat
Replicates and tiles a matrix to create a larger matrix. - rcond
Calculates the reciprocal condition number of a matrix. - return
Ends the execution of a function and returns control to the calling statement. - read
Reads data from a file. - save
Saves variables to a file. - scanf
Reads formatted data from a stream. - scatter
Creates a scatter plot. - semilogx
Creates a plot with a logarithmic x-axis and a linear y-axis. - semilogy
Creates a plot with a logarithmic y-axis and a linear x-axis. - sin
Calculates the sine of a number. - size
Returns the number of rows and columns in a matrix or vector. - sort
Sorts the elements of a vector or the rows of a matrix. For example, sort(v) returns a vector with the elements of v sorted in ascending order. - sound
Plays an audio signal. - sqrt
Calculates the square root. - string
Converts a string to a number. For example, str2num('123') returns the number 123. - subplot
Enables the creation of multiple plots in a single figure. For example, subplot(2,1,1) creates a layout for two plots, one above the other, and selects the first plot for subsequent drawing. - sum
Adds up the elements of a vector or matrix. For example, sum(v) returns the sum of the elements of the vector v, while sum(A) returns a vector with the sum of the elements of each column of the matrix A. - svd
Calculates the singular value decomposition of a matrix. - syms
Creates symbolic variables. - system
Executes a system command. - %t
Represents the Boolean value "True". - tan
Calculates the tangent of an angle. - tanh
Computes the hyperbolic tangent of a number. - title
Adds a title to a graph. - trace
Computes the trace of a matrix (the sum of the elements on the main diagonal). - transpose
Calculates the transpose of a matrix. - tril
Returns the lower triangular part of a matrix. - triu
Returns the upper triangular part of a matrix. - type
Returns the type of an entity. - testmatrix
Generates various types of test matrices. - toeplitz
Creates a Toeplitz matrix given a sequence. - timer
Measures the execution time of a code. - tic , toc
Measure the elapsed time between the execution of the two commands. - uicontrol
Creates a user control object in a figure. - uimenu
Creates a menu in a figure. - uiputfile
Opens a dialog box to select a file to save. - uigetfile
Opens a dialog box to select a file to open. - uigetdir
Opens a dialog box to select a directory. - uisetcolor
Opens a dialog box to select a color. - uisetfont
Opens a dialog box to select a font. - union
Finds the union of two sets. - unique
Finds unique elements in an array. - uint8
Convert to an 8 bit unsigned integer data type. - uint16
Convert to a 16 bit unsigned integer data type. - uint32
Convert to a 32 bit unsigned integer data type. - uint64
Convert to a 64 bit unsigned integer data type. - variance
Calculates the variance of a data set. - vectors
Creates a data vector. - verchol
Performs Cholesky decomposition on a variance matrix. - vertcat
Concatenates vertically two or more matrices or vectors. - view_pixmap
Displays a pixmap image. - viterbi
Implements the Viterbi algorithm. - vtranspose
Performs a vertical transformation of a matrix. - vsum
Performs the sum along the vector dimension. - voronoi
Calculates the Voronoi diagram. - vmd
Performs the variable mode decomposition. - warningThis function allows you to control how Scilab reacts when it encounters a warning during the execution of a command. You can use it to enable, disable, or customize warning messages.
- what
This function provides a list of all the functions available in the Scilab directories and module directories. - whos
This function provides a detailed list of all the variables currently in the working memory, showing their sizes and data types. - who
This function provides a list of all the variables currently in the working memory. - writemat
This function allows you to write a matrix or a set of matrices to a binary file. - write
This function allows you to write data to a file. - waitbar
This function creates a progress bar that can be used to indicate the progress of a long-lasting operation. - wavread , wavwrite
These functions are used to read and write WAV audio files. - xchange
Swaps a variable with another variable. - xclick
Waits for a mouse click in a graphic window and returns the coordinates. - xdel
Deletes a graphic window. - xend
Ends the drawing phase in a graphic window. - xfarc
Draws a full arc in a graphic window. - xget
Gets the value of a property of a graphic window. - xgrid
Adds a grid to a plot in a graphic window. - xlabel
Adds a label to the x-axis of a graph. - xlim
Sets the limits of the x-axis of a graph. - xset
Sets the value of a property of a graphic window. - zgrid
This function draws a grid on a Nyquist or Bode plot. - zeros
Creates a matrix of 0s. For example, zeros(2,2) creates a 2x2 matrix of 0s. - zeig
This function calculates the eigenvalues of a matrix. - zfrexp
Extracts the mantissa and exponent of a floating-point number. - zcos
Calculates the cosine of a complex number. - zsin
Calculates the sine of a complex number. - ztan
Calculates the tangent of a complex number. - zcosd
Computes the cosine of a complex number, but with the angle specified in degrees rather than radians. - zsind
Works out the sine of a complex number, but with the angle given in degrees rather than radians. - ztand
Finds the tangent of a complex number, but with the angle defined in degrees instead of radians. - zfrac
This function calculates the fraction of a number.