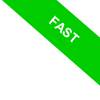
IF ELSE conditional structure in Matlab
Here we have an interesting topic to discuss: the IF ELSE conditional structure in Matlab.
What is a conditional structure? A conditional structure is a statement that allows you to execute a block of code only if a certain condition is met. In Matlab, to create a conditional structure, you use the if elseif else end statement.
To create a conditional structure in Matlab, you must use the if elseif else end instruction.
if main condition
code1
elseif condition2
code2
else
code3
end
Let me explain how it works.
When the main condition after the "if" statement is true, Matlab executes the first block of code (codice1) and exits the conditional structure.
If the main condition is false, Matlab moves on to check the next condition after the "else if" clause (condition2).
If this condition is true, it executes the second block of code (codice2) and exits the conditional structure.
Note. The else if clause is optional. You can include more than one or even none at all within the if end conditional structure.
If no condition is met, Matlab executes the block of code after the "else" clause (codice3).
At the end of the conditional structure, always remember to insert the keyword "end".
Note. It's worth noting that the "else if" clause is optional and you can include multiple clauses or none at all. The same goes for the "else" clause. You can create a conditional structure without including it.
Let me give you a practical example.
Consider this script that asks the user to input a number:
x=input("Enter a number ")
if (x==0)
disp("It's zero")
elseif (x==1)
disp("It's one")
elseif (x>0)
disp("It's a positive number")
else
disp("It's a negative number")
end
In this script, the program checks if the number input by the user is equal to zero.
- If it is, it prints "It's zero".
- If it's not equal to zero, it checks if it's equal to one and prints "It's one" if true.
- If it's not equal to one, it checks if it's greater than zero and prints "It's a positive number" if true.
Finally, if none of the above conditions are met, it prints "It's a negative number".
This is just one example of how you can use a conditional structure in Matlab.
Example 2
This script consists only of the if and else keywords.
x=input("Enter a number ")
if (x==0)
disp("It's zero")
else
disp("It's not zero")
end
The conditional structure checks if the number entered by the user is zero (if).
- If true, it prints "It's zero"
- If false (else), it prints "It's not zero"
In this case, there are two possible actions depending on the result.
Example 3
This script consists only of the main condition.
x=input("Enter a number ")
if (x==0)
disp("It's zero")
end
The conditional structure checks if the number is equal to zero.
- If true, it prints "It's zero"
- If false, it does nothing
In this case, there is only one possible action because the "else" clause is missing, and there are no "else if" clauses to check beyond the main condition.
You can create more complex structures by combining multiple conditions and clauses. The possibilities are endless!